The way my library has evolved is that it is split into basically 4 types of module: Implicit (multi-dimensional) noise sources, 2D Greyscale buffered sources, 2D RGBA buffered sources, and output modules.
The Implicit sources, as seen in the entries on Minecraft level generation, are a set of functions based on the generation of noise in 2, 3, 4 and 6 dimensions. This set includes noise sources, fractal combiners, domain transformations, all sorts of combiner modifiers, etc... Each function in this domain provides multi-dimensional output in order to facilitate the use of multiple dimensions for things such as animated noise and using 4 or 6 dimensions to create regular or seamless output as per the method I outlined in this earlier journal entry: Seamless Noise
2D Greyscale functions operate explicitly on allocated buffers of double floating point values. These include the same type of combiners and domain modifiers that implicit functions include (transforms, turbulence, etc) as well as their own set of pattern functions and generators. An adapter class exists to act as an interface with an implicit function; this adapter class allows the specification of how noise is mapped from an implicit generator to populate a 2D buffer, and parameters allow for the specification of different types of seamless mappings.
2D RGBA functions operate explicitly on allocated buffers of RGBA values, and include options for compositing different images together. Adapters are included to perform the mapping of 2D Greyscale data to RGBA in a variety of different ways, including color-scale mapping, alpha blending, HSV composition, etc...
Currently, the output domain only has a single module that takes as input a single RGBA module (the end of a chain) and evaluates it out to a .TGA file, allowing the specification of output file format and image dimensions.
In order to generate complex patterns, a chain of modules is specified. Previously, I was building such modules and chaining them by hand, calling constructors and setting sources in a process that was as icky and tedious as it sounds, but now that I have finally been implementing the simple system to allow me to specify them in a more concise table format as input to a parser that builds the chain automatically, the process has been simplified quite a bit, and it is easier to wrap my head around the complexity that some module trees represent.
Awhile back ( Here, in fact ) I did some tinkering with procedurally generated islands or continents. I haven't done anything with that in awhile, so to test out some of the new stuff I revisited those old techniques, using the new specification system to build a more complex module chain that can generate islands in a similar fashion to what I was doing before. Something like this could be useful, I think, for a Dwarf Fortress style exploration game, if the generator is fleshed out appropriately. I spent a couple hours this afternoon putting together a specification for a complex tree of modules to procedurally generate an island. Here are a couple images of some of the results:
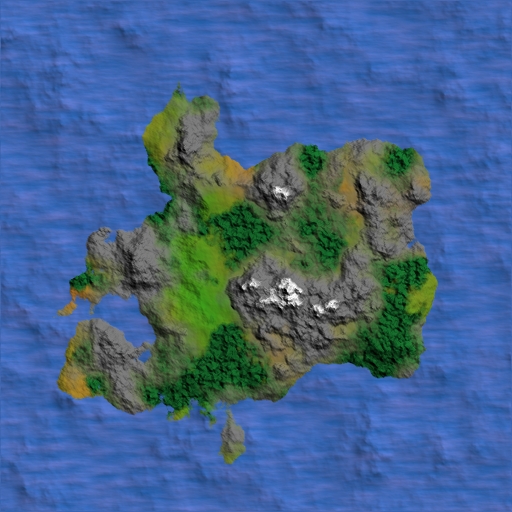
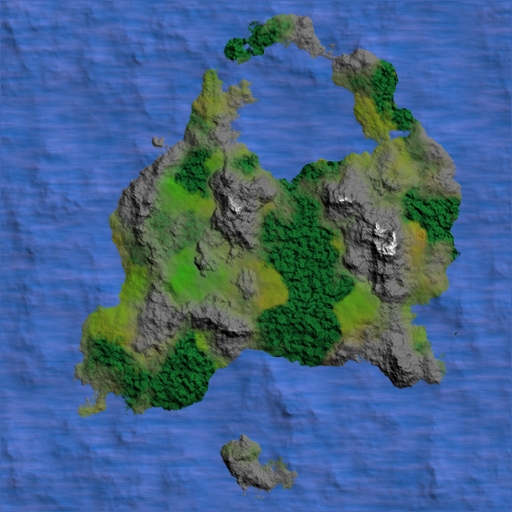
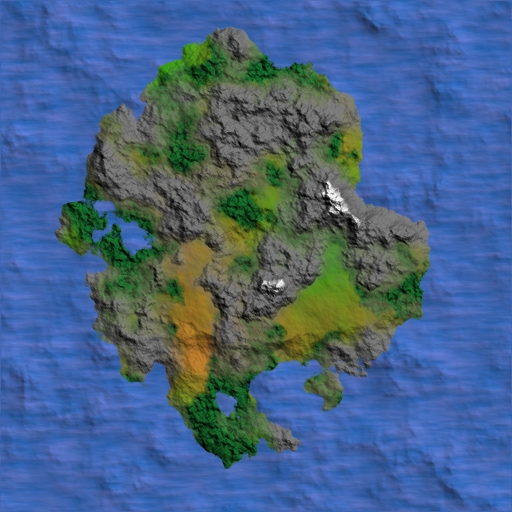
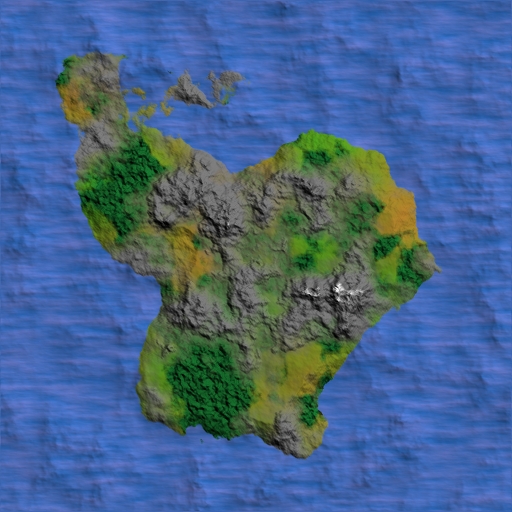
The tree is a bit more complex than the minecraft level generator in my previous post. It begins with a FuzzyDisk, which is just a pattern that uses the distance from center to assign a value to a cell in the buffer. This disk is then perturbed using some fractal functions to give it shape. Other fractals are set up to provide land forms: ocean floor, lowland plains and mountains are the only landforms currently, for simplicity. Yet other fractals are set up to provide blend masks between dirt->grass, stone->snow, and dirt/grass->forest, as well as lowlands->mountains. Modules are specified to provide bump-map relief information, to show the shape of the ground and to give the forested areas and ocean surface some texture, then a series of RGBA blend operations are set up, using the various fractals and masks, to create a colormap by selecting from seven colors: stone, snow, grass, dirt, forest, light water, dark water. The colormap is given value by multiplying it by the relief map, and the result is as you see in the above images. Given the relative quickness with which I put the module tree together for testing purposes, it is kind of messy but if you are interested the module chain is here:
testisland=
{
-- The main shape of the continent is founded on a fuzzy disk
{name="ContinentDisk", type="greyscale_fuzzydisk", radius=0.75, centerx=0.5, centery=0.5, seamless=false},
-- Add some X and Y axis turbulence to the fuzzy disk
{name="ContinentXTurb", type="greyscale_fractal", fractal_type="FBM", basis_type="GRADIENT", interp_type="QUINTIC",
frequency=3, num_octaves=10, remaprange=true, low=-1, high=1},
{name="ContinentYTurb", type="greyscale_fractal", fractal_type="FBM", basis_type="GRADIENT", interp_type="QUINTIC",
frequency=3, num_octaves=10, remaprange=true, low=-1, high=1},
{name="ContinentTurb", type="greyscale_turbulence", main_source="ContinentDisk", xaxis_source="ContinentXTurb", yaxis_source="ContinentYTurb",
xpower=100, ypower=100, seamless=true},
-- Remap the fuzzy disk function to the range [0,1]
{name="ContinentRemap", type="greyscale_remaprange", source="ContinentTurb", low=0, high=1},
-- Create a selection mask to outline the shape of the continent and blend between ocean floor and continent landforms.
{name="GConstant0", type="greyscale_constant", constant=0},
{name="GConstant1", type="greyscale_constant", constant=1},
{name="ContinentMask", type="greyscale_select", main_source="ContinentRemap", low_source="GConstant0", high_source="GConstant1",
threshold=0.3, falloff=0.15},
-- Create a selection mask to define the shoreline
{name="ShorelineMask", type="greyscale_select", main_source="ContinentRemap", low_source="GConstant0", high_source="GConstant1",
threshold=0.15, falloff=0.01},
-- Create a fractal for the ocean floor
{name="OceanFloorFractal", type="greyscale_fractal", fractal_type="HYBRIDMULTI", basis_type="GRADIENT", interp_type="QUINTIC",
numoctaves=8, frequency=6},
{name="OceanFloorRemap", type="greyscale_remaprange", source="OceanFloorFractal", low=0, high=0.3},
-- Create a fractal for mountains
{name="MountainFractal", type="greyscale_fractal", fractal_type="FBM", basis_type="GRADIENT", interp_type="QUINTIC",
numoctaves=10, frequency=22, remaprange=true, low=0.45,high=1},
-- Create a fractal for rolling hills
{name="RollingHillsFractal", type="greyscale_fractal", fractal_type="FBM", basis_type="GRADIENT", interp_type="QUINTIC",
numoctaves=4, frequency=6, remaprange=true, low=0.25, high=0.45},
-- Create a fractal mask to separate areas of mountain from areas of non-mountain
{name="MountainMaskFractal", type="greyscale_fractal", fractal_type="FBM", basis_type="GRADIENT", interp_type="QUINTIC",
numoctaves=4, frequency=6, remaprange=true, low=0, high=1},
{name="MountainMask", type="greyscale_select", main_source="MountainMaskFractal", low_source="GConstant0", high_source="GConstant1",
threshold=0.7, falloff=0.19},
-- Create a blender to combine mountains with lower terrain
{name="MountainLowlandBlend", type="greyscale_blend", main_source="MountainMask", low_source="RollingHillsFractal", high_source="MountainFractal"},
{name="ContinentBaseAdder", type="greyscale_combiner", combiner_type=0, source_0="ContinentBase", source_1="MountainLowlandBlend"},
-- Continent blend
{name="ContinentBlend", type="greyscale_blend", main_source="ContinentMask", low_source="OceanFloorRemap", high_source="MountainLowlandBlend"},
{name="ContinentBump", type="greyscale_bumpmap", source="ContinentBlend", sharpness=4, light={1.5,3.5,-1.5}},
-- RGBA Modules
{name="RGBAConstantBlueDark", type="rgba_constant", red=0.2, green=0.5, blue=0.9},
{name="RGBAConstantBlueLight", type="rgba_constant", red=0.5, green=0.6, blue=1},
{name="RGBAConstantGrey", type="rgba_constant", red=0.5, green=0.5, blue=0.5},
{name="RGBAConstantWhite", type="rgba_constant", red=1,green=1,blue=1},
{name="RGBAConstantBrown", type="rgba_constant", red=195/255, green=130/255, blue=46/255},
{name="RGBAConstantGreen", type="rgba_constant", red=0.2, green=0.7, blue=0.1},
{name="RGBAConstantDarkGreen", type="rgba_constant", red=0.1, green=0.5, blue=0.2},
-- Create a stretched ridged multi fractal to setup a wave pattern
{name="WaveRippleFractal", type="greyscale_fractal", fractal_type="FBM", basis_type="GRADIENT", interp_type="QUINTIC",
numoctaves=3, frequency=12, mapx1=0, mapy1=0, mapx2=1, mapy2=5, remaprange=true, low=0, high=1},
{name="WaveRippleBump", type="greyscale_bumpmap", source="WaveRippleFractal", sharpness=0.1, light={1.5,3.5,-1.5}},
{name="WaveLightColor", type="rgba_combinealpha", greyscale_source="WaveRippleFractal", rgba_source="RGBAConstantBlueLight"},
{name="WaveColor", type="rgba_blend", source1="WaveLightColor", source2="RGBAConstantBlueDark", blendop1=0, blendop2=2},
{name="RGBAWavePattern", type="rgba_multrgbagreyscale", greyscale_source="WaveRippleBump", rgba_source="WaveColor"},
-- Multiply the mountain elevation map by the mountain mask and the shoreline mask in order to get a "real" elevation map for
-- adding snow to higher elevation mountains,, then set up a selection buffer and do a blend between grey and white
{name="MountainFractalMultiply", type="greyscale_combiner", combiner_type=ECT_MULT, source_0="MountainFractal", source_1="MountainMask",
source_2="ContinentMask"},
{name="MountainSnowLineSelect", type="greyscale_select", main_source="MountainFractalMultiply", low_source="GConstant0", high_source="GConstant1",
threshold=0.65, falloff=0.075},
{name="MountainSnowLayer", type="rgba_combinealpha", greyscale_source="MountainSnowLineSelect", rgba_source="RGBAConstantWhite"},
{name="MountainSnowBlend", type="rgba_blend", source1="MountainSnowLayer", source2="RGBAConstantGrey", blendop1=0, blendop2=2},
-- Setup a fractal to blend between green and brown for lowland colors
{name="LowlandBlendMask", type="greyscale_fractal", fractal_type="FBM", basis_type="GRADIENT", interp_type="QUINTIC",
numoctaves=6, frequency=4, remaprange=true, low=0, high=1},
{name="LowlandColorLayer", type="rgba_combinealpha", greyscale_source="LowlandBlendMask", rgba_source="RGBAConstantGreen"},
{name="LowlandColorBlend", type="rgba_blend", source1="LowlandColorLayer", source2="RGBAConstantBrown", blendop1=0, blendop2=2},
-- Setup a tighter version of the mountain mask
{name="MountainColorMask", type="greyscale_select", main_source="MountainMaskFractal", low_source="GConstant0", high_source="GConstant1",
threshold=0.5, falloff=0.15},
-- Invert the mountain color mask, set up a forest fractal mask and multiply it by the mountain mask, ie no forest cover in mountains
{name="MountainColorMaskInvert", type="greyscale_remaprange", source="MountainColorMask", low=1, high=0},
{name="ForestBlendMask", type="greyscale_fractal", fractal_type="FBM", basis_type="GRADIENT", interp_type="QUINTIC",
numoctaves=6, frequency=6, remaprange=true, low=0, high=1},
{name="ForestBlendMaskSelect", type="greyscale_select", main_source="ForestBlendMask", low_source="GConstant0", high_source="GConstant1",
threshold=0.5, falloff=0.05},
{name="ForestMask", type="greyscale_combiner", combiner_type=ECT_MULT, source_0="ForestBlendMaskSelect", source_1="MountainColorMaskInvert"},
-- Setup a color blend layer for forest/lowland
-- First, set up a bump source to make bumpy forest color
{name="ForestBumpFractal", type="greyscale_fractal", fractal_type="FBM", basis_type="GRADIENT", interp_type="QUINTIC",
numoctaves=1, frequency=64, remaprange=true, low=0, high=1},
{name="ForestBump", type="greyscale_bumpmap", source="ForestBumpFractal", sharpness=1, light={1.5,3.5,-1.5}},
{name="ForestColorBump", type="rgba_multrgbagreyscale", greyscale_source="ForestBump", rgba_source="RGBAConstantDarkGreen"},
-- Now, blend in forest color
{name="ForestColorLayer", type="rgba_combinealpha", greyscale_source="ForestMask", rgba_source="ForestColorBump"},
{name="VegetationColorBlend", type="rgba_blend", source1="ForestColorLayer", source2="LowlandColorBlend", blendop1=0, blendop2=2},
{name="RGBAMountainLayer", type="rgba_combinealpha", greyscale_source="MountainColorMask", rgba_source="MountainSnowBlend"},
{name="RGBAMountainBlend", type="rgba_blend", source1="RGBAMountainLayer", source2="VegetationColorBlend", blendop1=0, blendop2=2},
-- Blend between land colors and ocean colors using the shoreline mask
{name="RGBALandMap", type="rgba_combinealpha", greyscale_source="ShorelineMask", rgba_source="RGBAMountainBlend"},
{name="RGBACombineLandOcean", type="rgba_blend", source1="RGBALandMap", source2="RGBAWavePattern", blendop1=0, blendop2=2},
-- Multiple the whole thing by the elevation bump-map
{name="RGBACombineBump", type="rgba_multrgbagreyscale", greyscale_source="ContinentBump", rgba_source="RGBACombineLandOcean"},
{name="RGBAAdapter", type="rgba_greyscaleadapter", source="MountainFractalMultiply"},
{name="Output", type="output", source="RGBACombineBump", mode=0, width=512, height=512}
}
And if you are masochistic and would like to experiment with it, maybe tweak a few parameters, an experimental framework package is here:
EDIT: The previous version of the script had a bug in that the num_octaves of several fractals was incorrectly specified, this one should be fixed.
Just execute the Lua interpreter and do the command dofile("testmodules.lua") in order to parse the table, seed the generators, and output a TGA called test.tga.
Great job on these, im learning quite a bit