When you say 'point' in your algorithm you mean vertex and not mid-point right? From your description your algorithm should work.
Try this:
- for every possible edge calculate its circumcircle (find the midpoint as you already have and then the radius)
- throw away every edge with more than 0 vertices in its circumcircle (use < and not <= as your original two points should be equal/on the circumcircle, or explicitly exclude the original two, also floating point math may mean you might need a small 'fudge'/epsilon factor here)
- create your triangles from the remaining edges
I think that should work. Or perhaps I hope
I feel like I am getting close! I am getting some funky spaces in the middle of bunches of triangles though. What do you think is causing this?
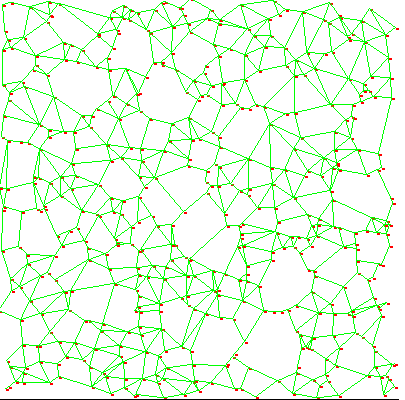
Here's the code I am using to solve for edges [my apologies ahead of time for lack of elegance]:
//Make Edges
for(int a=0;a<pA.size();a++)
{for(int b=a+1;b<pA.size();b++){
eA.add(new Edge(pA.get(a),pA.get(b)));
}}
//Cull Edges
ArrayList<Edge> cull = new ArrayList<Edge>();
for(int e=0;e<eA.size();e++)
{for(int p =0;p<pA.size();p++){
if(!eA.get(e).remove&&eA.get(e).center.getDistanceSquare(pA.get(p))<eA.get(e).radiusSquare){
eA.get(e).remove=true;
cull.add(eA.get(e));
}}}
for(int c=0;c<cull.size();c++)
{eA.remove(cull.get(c));
}