Hi, i've been adding directional lightmaps to an old DX9 engine, which is working fine, but i wanted to have a go at adding specular highlights for static lighting. I've heard that other engines such as Unity and UE3 have this but my google-fu is failing me as I can't find any solid implementation details. So, i've had a blind stab at it myself but there's a divide by zero issue blocking me at the moment.
The theory is that the directional lightmaps have an approximation of the light hitting a surface, so rather than getting the diffuse light by using the surface normal directly (with normal map) I get the reflected light by using the reflected eye vector. I then use the luminance of the reflected light as it's attenuation, on which the specular exponent and coefficient is applied before multiplying the reflected light to get the final specular value.
This doesn't seem to work too badly, even if it is totally innacurate and bodged together
.

Diffuse light

Specular Light

Specular attenuated

Baseline (No directional lightmaps)
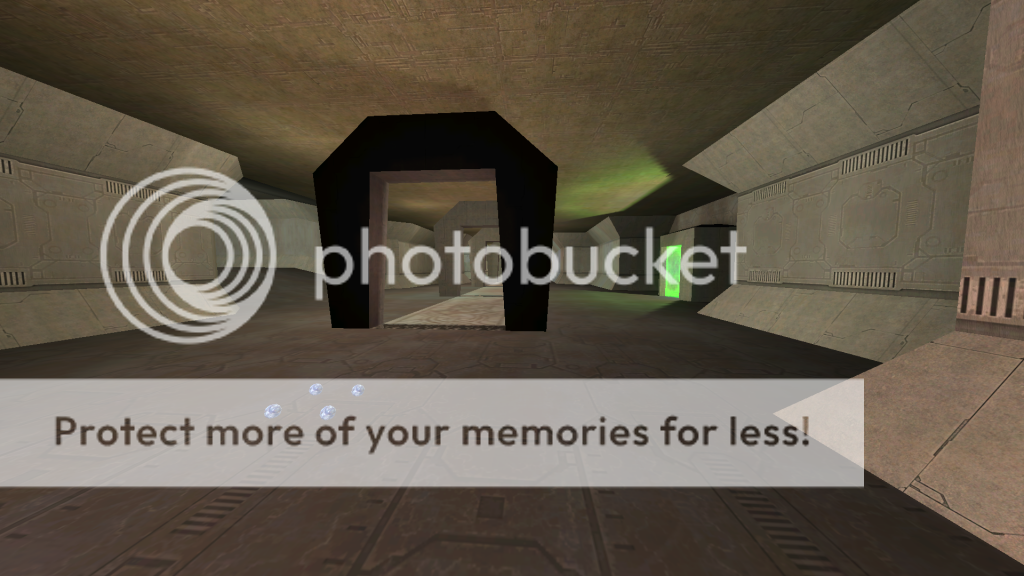
Final Diffuse

Final Diffuse + Specular

Code:
static const float3 BumpBasis[3] =
{
float3( sqrt(2.0) / sqrt(3.0), 0.0, 1.0 / sqrt(3.0)),
float3( -1.0 / sqrt(6.0), 1.0 / sqrt(2.0), 1.0 / sqrt(3.0)),
float3( -1.0 / sqrt(6.0), -1.0 / sqrt(2.0), 1.0 / sqrt(3.0))
};
float3 CalculateDLMContribution(float3 TangentNormal)
{
TangentNormal.y = -TangentNormal.y;
float3 lightmapContribution = 0;
lightmapContribution.x = dot(BumpBasis[0], TangentNormal);
lightmapContribution.y = dot(BumpBasis[1], TangentNormal);
lightmapContribution.z = dot(BumpBasis[2], TangentNormal);
lightmapContribution = saturate(lightmapContribution);
lightmapContribution *= lightmapContribution;
return lightmapContribution;
}
float3 CalculateDLMDiff(float3 TangentNormal,
float3 DLightmap0,
float3 DLightmap1,
float3 DLightmap2)
{
float3 lightmapContribution = CalculateDLMContribution(TangentNormal);
float3 light = 0;
float sum = dot(lightmapContribution, float3(1.0f, 1.0f, 1.0f));
light += DLightmap0 * lightmapContribution.x;
light += DLightmap1 * lightmapContribution.y;
light += DLightmap2 * lightmapContribution.z;
light /= sum;
return light;
}
float3 CalculateDLMSpec(float3 TangentNormal,
float3 EyeVectorTS,
float3 DLightmap0,
float3 DLightmap1,
float3 DLightmap2)
{
float3 reflectedEye = -reflect(normalize(EyeVectorTS), TangentNormal);
float3 lightmapContribution = CalculateDLMContribution(reflectedEye);
float3 light = 0;
light += DLightmap0 * lightmapContribution.x;
light += DLightmap1 * lightmapContribution.y;
light += DLightmap2 * lightmapContribution.z;
light /= 1.33f; // Arbitrary value :(
float luminance = dot(light, float3(0.30f, 0.59f, 0.11f));
luminance = pow(luminance, 6.0f) * 7.0f; // Hardcoded test values
return saturate(light * luminance);
}
However, the issue I mentioned is with the light averaging that occurs in the diffuse light calculation. I'd expect to have to do the same in the specular light calculation but if I do I end up with a divide by zero since the reflected vector can point below the surface normal resulting in zero light contributions from any of the 3 basis directions. This causes a harsh black spot in the resulting specular light, and i'm not sure how to counter it.
Black Spots
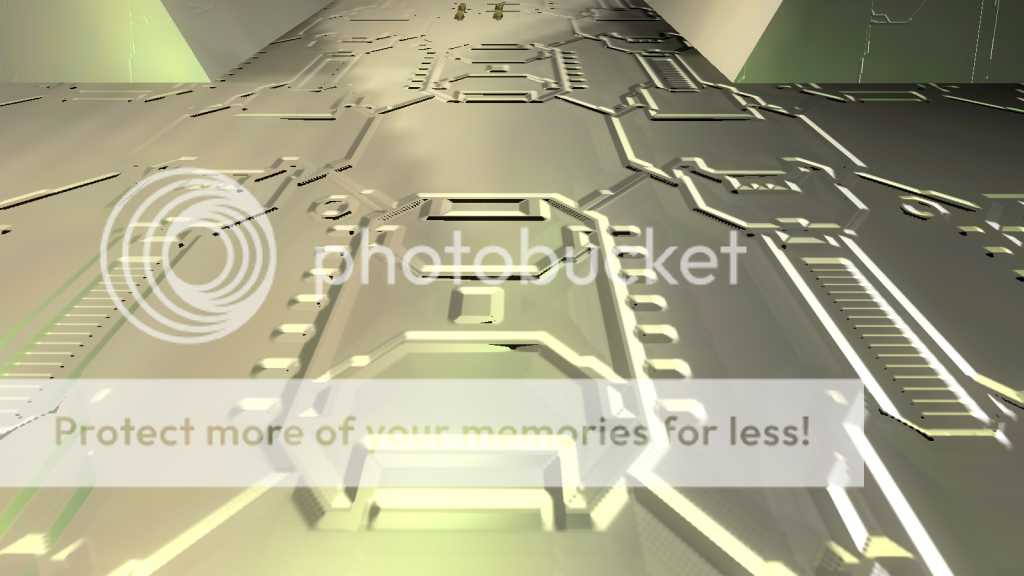
Highlighted

At the moment i'm dividing by an arbitrary value to get a reasonable result but i'm hoping there's a *proper* way to handle this?