Hello, i'm trying to write a simple 3d software renderer in c# .net but have run into problems with the matrices/projection part.
Below i have included the relevant part of my code, it's capable of projecting a triangle in 3d space and then rasterize it
public void RenderTriangle(System.Numerics.Vector4 v1, System.Numerics.Vector4 v2, System.Numerics.Vector4 v3, Color4 color, Camera camera, float interaction)
{
// create matrices
var viewMatrix = Matrix4x4.CreateLookAt(new System.Numerics.Vector3(camera.Position.X, camera.Position.Y, camera.Position.Z), new System.Numerics.Vector3(camera.Target.X, camera.Target.Y, camera.Target.Z), System.Numerics.Vector3.UnitY);
var modelMatrix = Matrix4x4.CreateFromYawPitchRoll(0, 0, 0) * Matrix4x4.CreateTranslation(0f, 0f, 0f);
var transformMatrix = modelMatrix * viewMatrix;
var projectionMatrix = Matrix4x4.CreatePerspectiveFieldOfView(0.78f, (float)Width / Height, 0.01f, 1000.0f);
// transform modelview
var transformed1 = System.Numerics.Vector4.Transform(v1, transformMatrix);
var transformed2 = System.Numerics.Vector4.Transform(v2, transformMatrix);
var transformed3 = System.Numerics.Vector4.Transform(v3, transformMatrix);
// transform projection
var fv1 = System.Numerics.Vector4.Transform(transformed1, projectionMatrix);
var fv2 = System.Numerics.Vector4.Transform(transformed2, projectionMatrix);
var fv3 = System.Numerics.Vector4.Transform(transformed3, projectionMatrix);
// perspective divide
fv1.W = 1f / fv1.W;
fv1 = new System.Numerics.Vector4(fv1.X * fv1.W, fv1.Y * fv1.W, fv1.Z * fv1.W, fv1.W);
fv2.W = 1f / fv2.W;
fv2 = new System.Numerics.Vector4(fv2.X * fv2.W, fv2.Y * fv2.W, fv2.Z * fv2.W, fv2.W);
fv3.W = 1f / fv3.W;
fv3 = new System.Numerics.Vector4(fv3.X * fv3.W, fv3.Y * fv3.W, fv3.Z * fv3.W, fv3.W);
//viewspace transform
fv1.X = fv1.X * Width + Width / 2.0f;
fv1.Y = -fv1.Y * Height + Height / 2.0f;
fv2.X = fv2.X * Width + Width / 2.0f;
fv2.Y = -fv2.Y * Height + Height / 2.0f;
fv3.X = fv3.X * Width + Width / 2.0f;
fv3.Y = -fv3.Y * Height + Height / 2.0f;
//
// 1. sort vertices based on winding order and y
// 2. line drawing algorithm to populate minx maxx arrays for each line for each y
// 3. go over each minx maxx and draw line
// 4. profit
}
mera = new Camera();
mera.Position = new Vector3(0, 0, 3.0f);
mera.Target = new Vector3(0, 0f, 0f);
TheDevice.RenderTriangle(new System.Numerics.Vector4(0.0f, 0.5f, 0f, 1f), new System.Numerics.Vector4(0.5f, -0.5f, 0f, 1f), new System.Numerics.Vector4(-0.5f, -0.5f, 0f, 1f), new Color4(1f, 1f, 1f, 1f), mera, interaction);
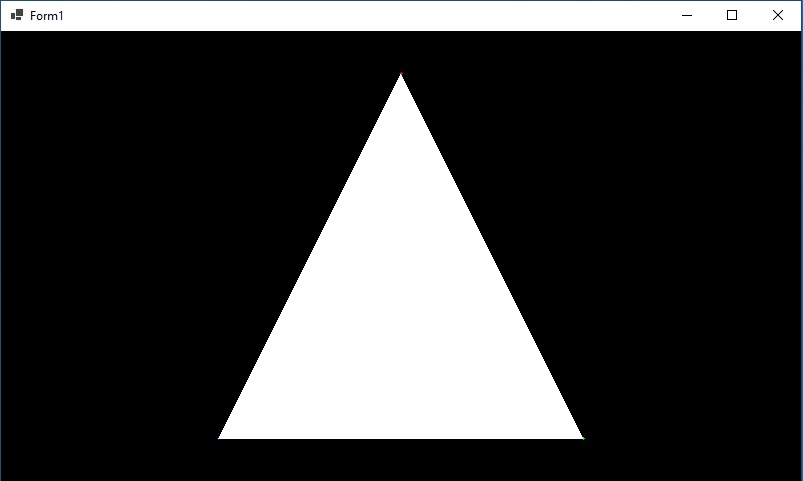
So far so good. The next two steps is clipping and texture mapping/colors interpolated across the triangle and herein lies my problem, this is the vertex pipeline for opengl https://www.scratchapixel.com/images/upload/perspective-matrix/vertex-transform-pipeline.png, have i gotten the pipeline right? is my code between “//transform projection” and “//perspective divide” the homogenous clip space? and is the w of my v1 v2 v3 the correct one after my transforms that i must divide with for perspective correct texturing? My knowlede of matrices is superficial and gained from playing with opengl where these things are taken care of behind the scenes, i only really understand that matrices move coordinates from one space into another.
I've used the built in System.Numerics vector and matrix structs, the code for them is as such:
The Matrix4x4 uses a right handed coordinate system where z moves towards you, and is row major.
Would very much appreciate any advice or help.