I have a 2:1 isometric 3D world where all of the world objects have AABBs, and I'm trying to add mouse selection of those objects. Positive X points to the bottom right, and positive Y points to the bottom left.
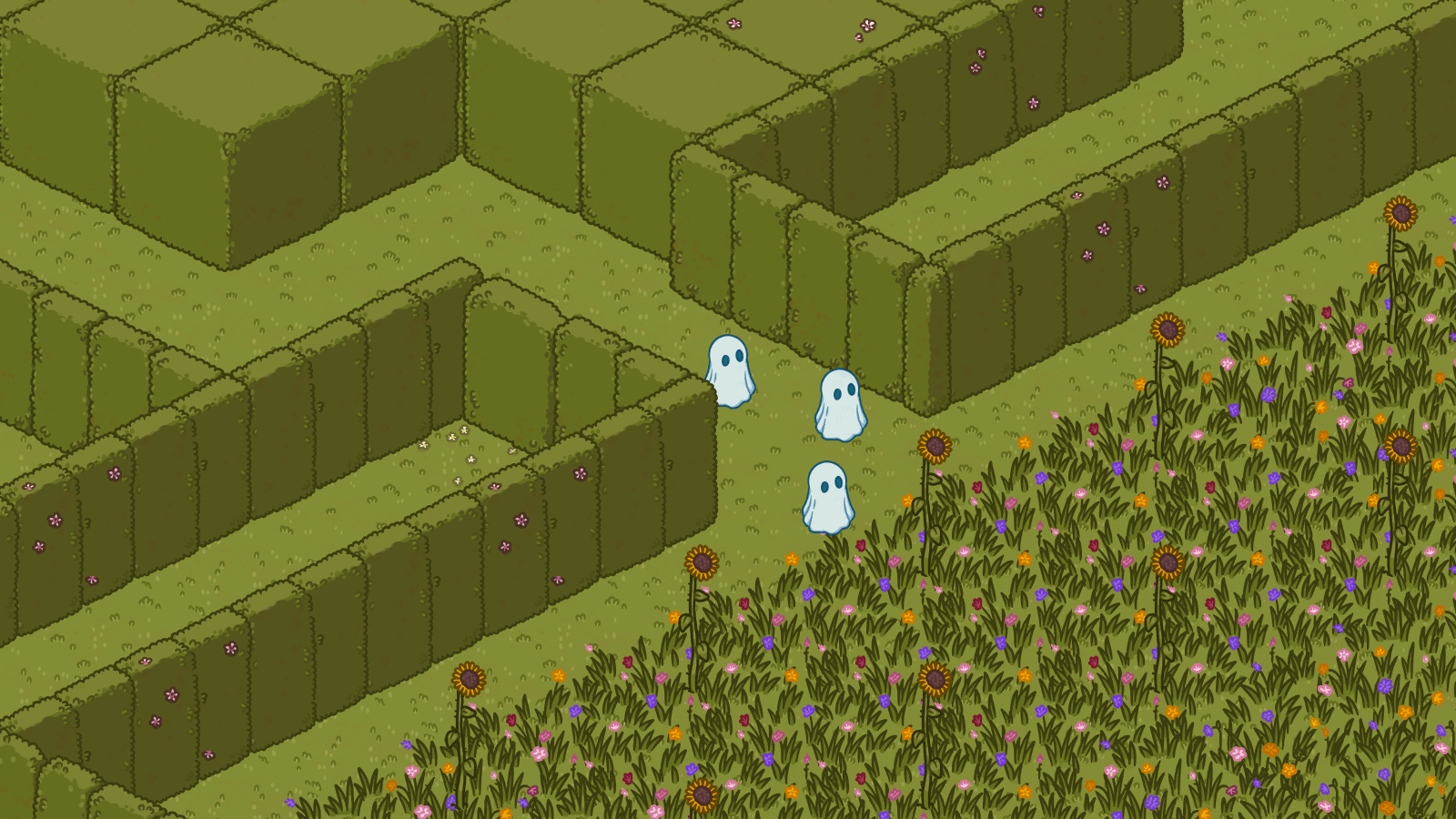
Where I'm at so far:
- From my rendering logic, I already have a list of all of the objects and their AABBs, sorted by their draw order.
- I can add this sorted list of objects to a spatial acceleration structure, to reduce the number of checks needed.
- I'm able to click a point on the screen and get the floor position (z == 0) that was clicked.
- I have some idea of how I could calculate the direction vector for this camera (rotate 60 around X, rotate -45 around Z).
- I think I could use the floor position + inverted direction vector to cast a ray from the floor, towards the camera. I could then ask the acceleration structure for the objects in the intersected tiles, then reverse-iterate them and check for intersections with the ray.
This seems like it would work, but I was wondering: is there a way to know the 3D position of a point on the screen, so I can just cast a ray from it instead of from the ground?
The reason why this is difficult is that my camera is very simple, and isn't already set up to itself have a position. Here's how it currently works:
- Set the world floor position that you want to center the camera on.
- Convert the world position to a point in isometric screen space.
- Use the point as the center of the camera, render anything within (point.x - w/2, point.y - h/2, w, h).
Since I'm just centering on a world position and doing the rest in screen space, I don't have any intuition about where the camera is actually located in the world.
Is there a straightforward way to do this? Should I forget it and just cast the ray from the floor? Is there a simpler overall approach that I should know about? Any help is appreciated!