Hi, I just wrote my first ray tracer using DX12 DXR. Everything checks out, doesn't produce error when debugged yet it doesn't render my model on screen. Here are some screen shots. Q1: Is it a must to have an index buffer of the model when we create accel. struct.? only difference between my implementation and Microsoft's sample cube rendering. My resource loader doesn't create an index buffer only the vertex buffer. I pass the vertex buffer as a structured buffer.
void VertexBufferView(ComPtr<ID3D12Device5> inDevice, ComPtr<ID3D12DescriptorHeap> mSrvHeap)const
{
D3D12_SHADER_RESOURCE_VIEW_DESC srvDesc = {};
srvDesc.ViewDimension = D3D12_SRV_DIMENSION_BUFFER;
srvDesc.Shader4ComponentMapping = D3D12_DEFAULT_SHADER_4_COMPONENT_MAPPING;
srvDesc.Buffer.FirstElement = 0;
srvDesc.Buffer.NumElements = mMeshGeometry.size();
srvDesc.Format = DXGI_FORMAT_UNKNOWN;
srvDesc.Buffer.Flags = D3D12_BUFFER_SRV_FLAG_NONE;
srvDesc.Buffer.StructureByteStride = VertexByteStride;
CD3DX12_CPU_DESCRIPTOR_HANDLE srvDescriptorHandle(mSrvHeap->GetCPUDescriptorHandleForHeapStart());
inDevice->CreateShaderResourceView(Mesh_Vertex_Buffer_GPU.Get(), &srvDesc, srvDescriptorHandle);
}
void CreateMeshVertexBuffer(ComPtr<ID3D12Device5> inDevice, ComPtr<ID3D12GraphicsCommandList5> inCommandList)
{
const UINT vbByteSize = (UINT)mMeshGeometry.size() * sizeof(RE_VERTEX);
Mesh_Vertex_Buffer_GPU = Common::CreateDefaultBuffer(inDevice.Get(),
inCommandList.Get(), mMeshGeometry.data(), vbByteSize, Mesh_Vertex_Buffer_Uploader);
VertexByteStride = sizeof(RE_VERTEX);
VertexBufferByteSize = vbByteSize;
}
...............................................
void RESrcLoader::BuildAccelerationStructures()
{
D3D12_RAYTRACING_GEOMETRY_DESC geometryDesc = {};
geometryDesc.Type = D3D12_RAYTRACING_GEOMETRY_TYPE_TRIANGLES;
geometryDesc.Triangles.Transform3x4 = 0;
geometryDesc.Triangles.VertexFormat = DXGI_FORMAT_R32G32B32_FLOAT;
geometryDesc.Triangles.VertexCount = static_cast<UINT>(Model[4].mMeshGeometry.size());
geometryDesc.Triangles.VertexBuffer.StartAddress = Model[4].Mesh_Vertex_Buffer_GPU->GetGPUVirtualAddress();
geometryDesc.Triangles.VertexBuffer.StrideInBytes = sizeof(RE_VERTEX);
geometryDesc.Triangles.IndexCount = 0;
geometryDesc.Triangles.IndexFormat = DXGI_FORMAT_UNKNOWN;
geometryDesc.Triangles.IndexBuffer = NULL;
................................................
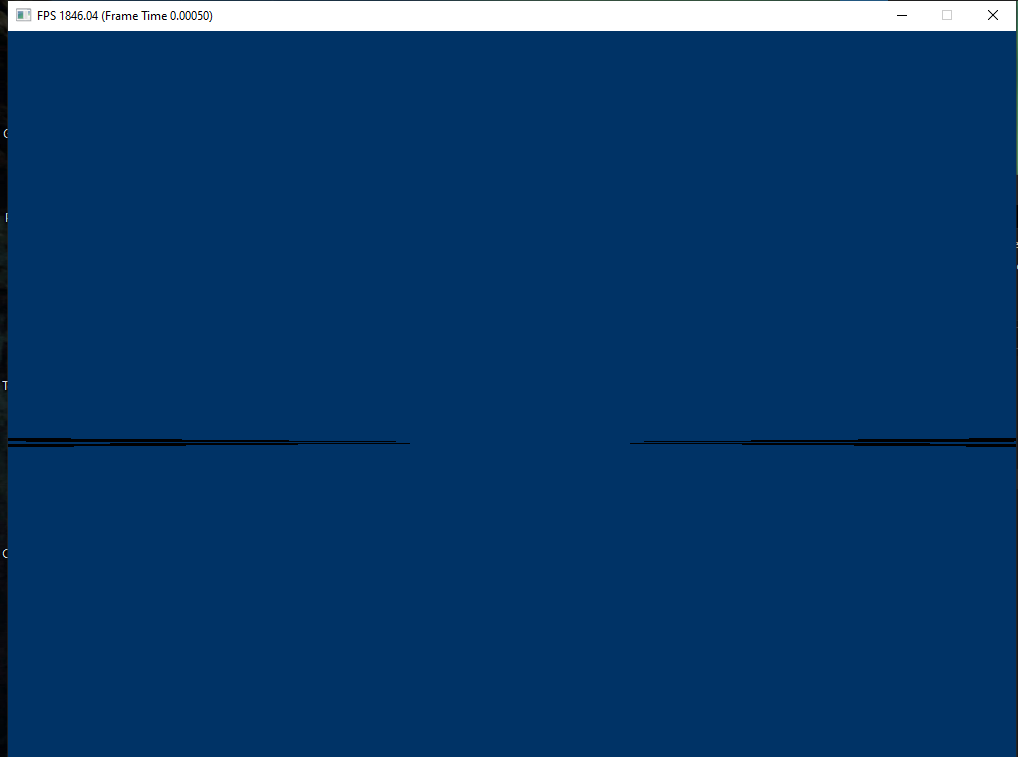
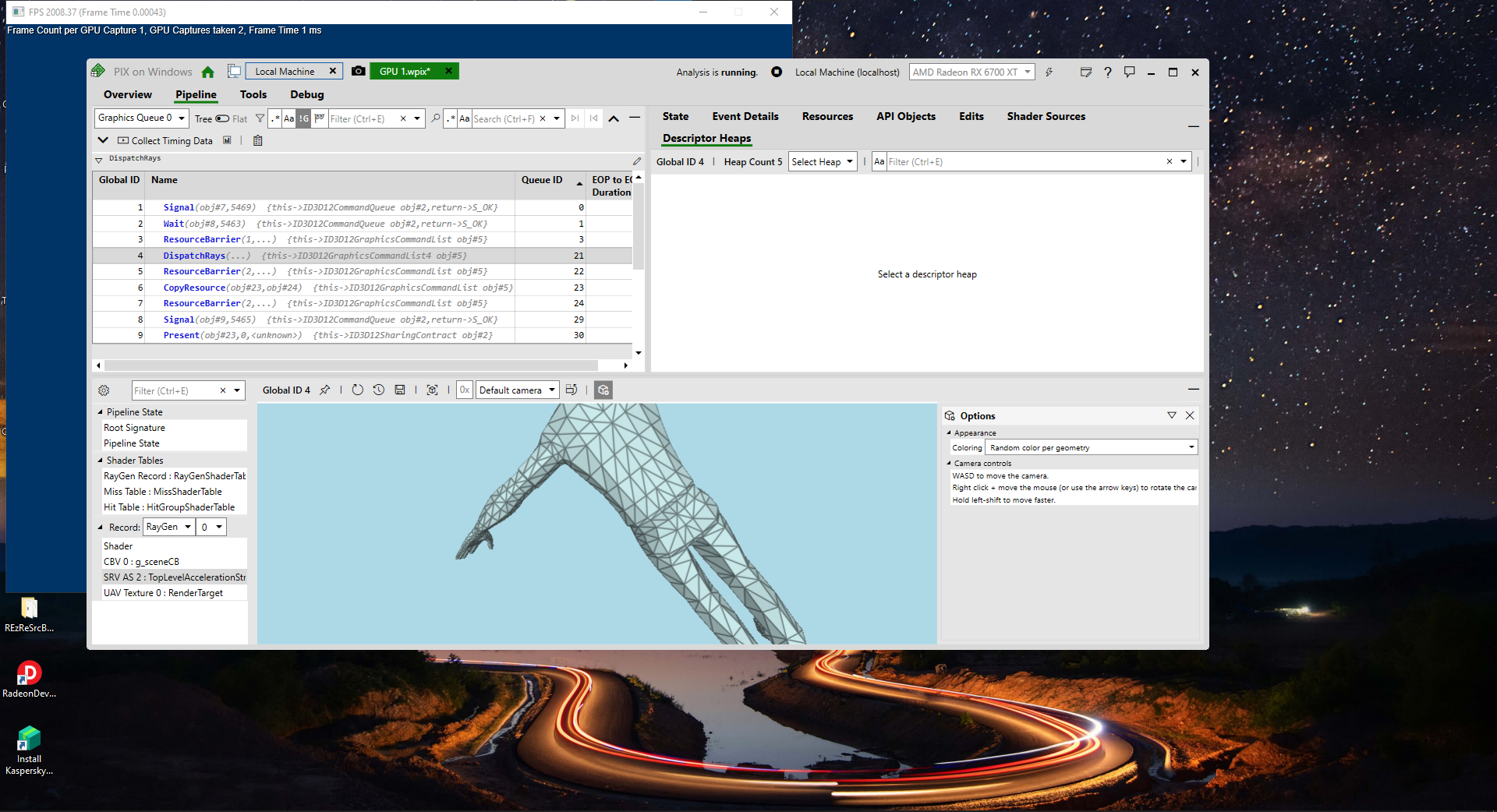
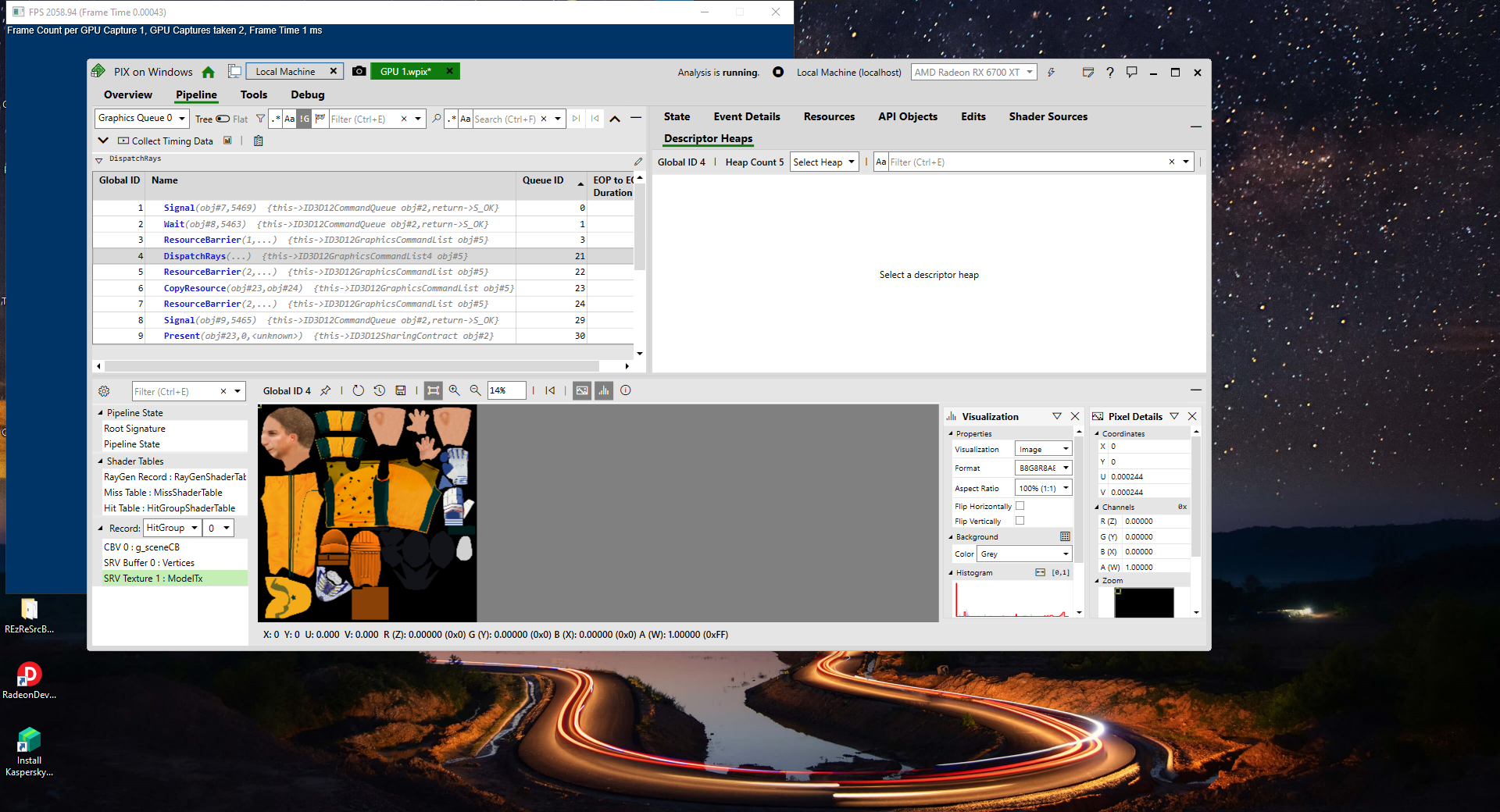