I'm working on some simple collision detection for a 2D game in XNA 3.1.
I'd like to know when a bullet object collides with a terrain object.
-My bullet textures are simple lines but they can be rotated.
-My terrain textures are small but irregular hills.
Currently, my rough collision detection is comparing two rectangles for intersection but that can look a bit weird if a bullet impacts a low spot in the terrain.
My current thought is to look for the intersection between rectangles. If that returns true, then I'd do a pixel by pixel comparison to see if any pixels are overlapping. This seems to be a nasty, nasty method since it's so computationally expensive.
My calculus inspired alternative is to break the terrain textures up into smaller textures and use the current rectangular collision detection. If the rectangles are divided into small enough blocks, I can believably get by. But the number of collision checks increases exponentially.
The last alternative is to try to create a polygon collision area which would outline the terrain contours... I don't know how to do that, so it'd take a bit of R&D to get it right.
What's the best practice for collision detection with irregularly shaped objects like terrain?
Collision Detection with irregularly shaped objects
I'm working on some simple collision detection for a 2D game in XNA 3.1.
I'd like to know when a bullet object collides with a terrain object.
-My bullet textures are simple lines but they can be rotated.
-My terrain textures are small but irregular hills.
Currently, my rough collision detection is comparing two rectangles for intersection but that can look a bit weird if a bullet impacts a low spot in the terrain.
My current thought is to look for the intersection between rectangles. If that returns true, then I'd do a pixel by pixel comparison to see if any pixels are overlapping. This seems to be a nasty, nasty method since it's so computationally expensive.
My calculus inspired alternative is to break the terrain textures up into smaller textures and use the current rectangular collision detection. If the rectangles are divided into small enough blocks, I can believably get by. But the number of collision checks increases exponentially.
The last alternative is to try to create a polygon collision area which would outline the terrain contours... I don't know how to do that, so it'd take a bit of R&D to get it right.
What's the best practice for collision detection with irregularly shaped objects like terrain?
if each bullet is a line, you can do line segment-line segment collision or even line pixel collision very cheaply.
...
I'd like to know when a bullet object collides with a terrain object.
-My bullet textures are simple lines but they can be rotated.
Rotated compared to what? The line of shot, or the fix world co-ordinate axes?
-My terrain textures are small but irregular hills.
Is it top-down? What means irregular (do you have a screen shot)? Which information describing the hills are available?
Currently, my rough collision detection is comparing two rectangles for intersection but that can look a bit weird if a bullet impacts a low spot in the terrain.
...
At least a line-rectangle test seems me possible.
'slayemin' said:…
I'd like to know when a bullet object collides with a terrain object.
-My bullet textures are simple lines but they can be rotated.
Rotated compared to what? The line of shot, or the fix world co-ordinate axes?
-My terrain textures are small but irregular hills.
Is it top-down? What means irregular (do you have a screen shot)? Which information describing the hills are available?
Currently, my rough collision detection is comparing two rectangles for intersection but that can look a bit weird if a bullet impacts a low spot in the terrain.
…
At least a line-rectangle test seems me possible.
Here is a screenshot. I added the red rectangles to show you the bounding rectangle borders.
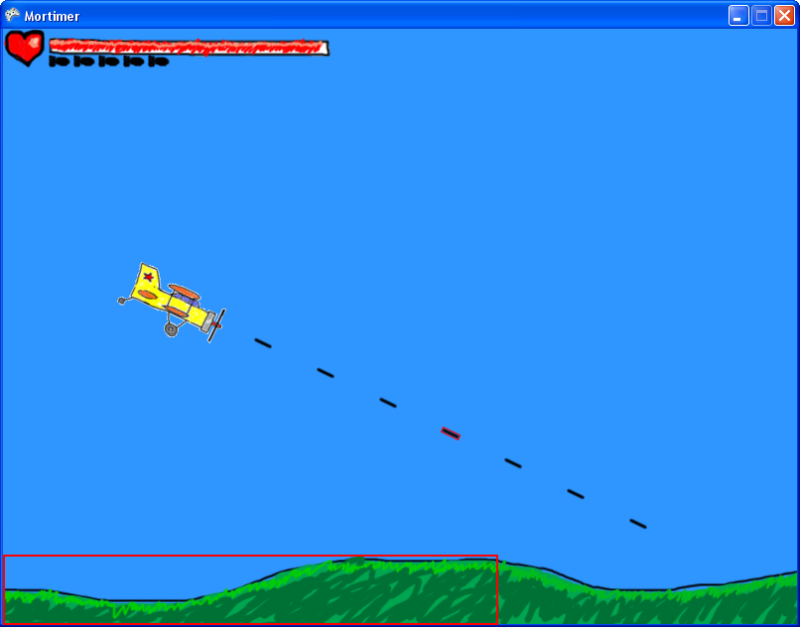
In this situation I would say that piecewise fixed heights (i.e. a series of screen aligned, more-or-less small rectangles) should be used for the ground, and a line for the shot. Pick a point of the shot, perhaps the one "in front", and use its horizontal co-ordinate to determine over which ground rectangle the shot currently is. When you use a regular grid for the ground rectangles (i.e. they are all of the same width), then you can compute the rectangle's index as simple as
i := ( shotx - offsetx ) / width
where offsetx can be used to consider an offset from the shot's origin to the origin from where the ground rectangles' positions are measured. If you use a non-regular grid then you need to do a search.
Then compare the vertical co-ordinate of the shot with the top edge co-ordinate of the previously determined ground rectangle. If the shot is above, then you're done. If it is below, then it already hit the ground. Something like this.
Of course, you can also make the width of the rectangles as small as 1 pixel, so that you can compare with a "ground mask", too.
i := ( shotx - offsetx ) / width
where offsetx can be used to consider an offset from the shot's origin to the origin from where the ground rectangles' positions are measured. If you use a non-regular grid then you need to do a search.
Then compare the vertical co-ordinate of the shot with the top edge co-ordinate of the previously determined ground rectangle. If the shot is above, then you're done. If it is below, then it already hit the ground. Something like this.
Of course, you can also make the width of the rectangles as small as 1 pixel, so that you can compare with a "ground mask", too.
Do you really need a line? It looks like a point to me. Because it is always the tip, that reaches the target first. So it is really a point. I'd go with a per-pixel collision, or maybe per 2x2 or 3x3 pixels. Store the map as a 2D array (the circular buffer or what is the name...) by "rasterizing" the map to 1x1 / 2x2 or whatever blocks, then you only have to update the parts that get outside/inside the view. It should be very cheap, even without any optimizations (like quad-tree or whatever)
you could pretty easily create a height map(well thats how i would explain it) , compare to see if the bullet is below the height of that X position, and do your stuff
ill draw an example on that screeny in a sec
ill draw an example on that screeny in a sec
where W= the width between the height map segments , and F=floor(bullet.x/W) , and assuming height map is stored in an array nammed "Hmap"
if(bullet.y>(Hmap[F]+(((Hmap[F]-Hmap[F+1])/W)*(bullet.x-(F*W)))) //(not sure if this equasion is correct, sorry for all the brackets lol)
do stuff
if(bullet.y>(Hmap[F]+(((Hmap[F]-Hmap[F+1])/W)*(bullet.x-(F*W)))) //(not sure if this equasion is correct, sorry for all the brackets lol)
do stuff
The problem with heightmaps that it can't have holes/caves/etc. That is one reason I don't like most of new scorched earth clones.
from what i can see it doesnt look like it has any holes/caves
besides even if there were you can still do it with a bit more math
besides even if there were you can still do it with a bit more math
This topic is closed to new replies.
Advertisement
Popular Topics
Advertisement