Introduction
This is not a step by step tutorial about coding.
I am just giving a script that you may find useful and can directly (Copy paste) use in any of your projects.
I expect that you keep a copyright reference to me and you can use the script freely.
Remember us!
Instead of reading, you may copy paste the script and use it in your project. However it would be good idea to directly jump to how to use this script.
Mounting Script
In any 3d Game Editor, the number objects in the scene increase rapidly during development. This lead to a lot of problems such as complicated clutter of hierarchy for objects inside object. If Unity3d prefabs are considered, then there maybe a need arising where developers my have to put prefabs inside prefabs and the design spaghetti rapidly grows.
Game Developers, designers and programmers work with such game objects which had 1000s of child objects. They consisted of empty objects, meshes, renders, colliders and scripts which is well managed but a time consuming activity in itself, even though it looked more like clutter, a well organized one. When the projects are long hauls, its easily forgotten where a object exits. Search helps but its limited. Especially after long lost bugs taken to task, it feels a big jungle.
There are two forms of problems of complex objects. a) During Editing. b) During Runtime. The details of these is not the scope of this tutorial. >:-o
Even though this script looks simple but depending upon its application this script can do a lot of heavy work during runtime. It can help in reducing the hierarchy of any 3d/2d object. I am sure experienced developers will understand.
The main use is objects will have simpler structure and easy to manage.
How to use the Mounting Script
Please take a look at the screenshot of the Inspector for the properties of script.
Some basic steps will follow after this screenshot. Its pretty simple.
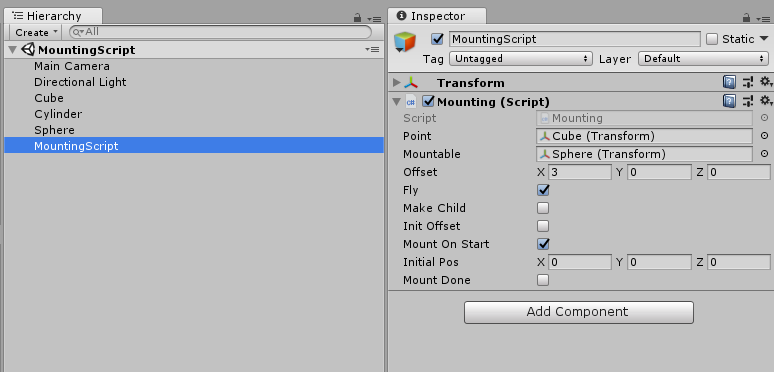
Some Basic Steps:
- Remember that, Mountable will attach to the Point. That all there is.
- The minimum requirement to start this script is that:
- You need to have two GameObjects,
- Point and,
- Mountable.
- Select either of the checkboxes Fly or MakeChild.
- Mount on start should always be ON.
- You need to have two GameObjects,
Some more info:
- Point : This is where the parent transform is placed.
- Mountable : Transform of the child.
- Offset : A safe distance between Point and Mountable.
- Fly : Do child parent without actually making a child parent inside the transform or GameObject.
- MakeChild : This is the preferred one, puts the mountable as child inside the Point.
- Init Offset: This will input the value of Offset. Otherwise offset is not used.
- Mount On Start : Mounting is initialized at the start.
- Initial Pos [Read Only]: Its a read only variable for storing the value of initial position of Mountable.
- Mount Done: Just a variable to view if Mount is done. Dont change it directly in editor.
Important Note:
The mounting can be once started and stopped as and when the programmer requires it. Use a call to method public void Mount() to mount and a call to Dismount() for dismounting the Mountable object.
The script Code: Just copy this code.
Just create a new script in unity3d and copy paste this code. That should get you started.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
/// <summary>
/// Author: Saurabh Torne
/// Created 07 Nov 2019
/// Copyright © 2019 Saurabh Torne.
/// Published on Gamdev.net.
/// Mounting and unmounting of items.
/// </summary>
public class Mounting : MonoBehaviour
{
public Transform Point, Mountable;
public Vector3 offset;
public bool fly = false;
public bool MakeChild = false;
public bool InitOffset = false;
public bool MountOnStart = true;
public Vector3 InitialPos;
public bool MountDone = false;
private
// Use this for initialization
void Start()
{
if (MountOnStart)
{
Mount();
}
}
// Update is called once per frame
void Update()
{
if (!MakeChild && MountDone)
{
Mountable.position = Point.position + offset;
Mountable.rotation = Point.rotation;
}
}
public void Mount()
{
InitialPos = Mountable.position;
if (InitOffset)
{
offset = Mountable.position - Point.position;
}
Mountable.position = Point.position + offset;
if (fly)
{
Mountable.parent = null;
MakeChild = false; // Important
}
else if (MakeChild)
{
Mountable.parent = Point;
}
else
{
return;
}
MountDone = true;
}
public void Dismount()
{
if (Mountable.parent == Point)
{
Mountable.parent = null;
}
MountDone = false;
}
}
This script has be very useful to me, hope that its useful to all.