Introduction
The technique presented here runs at 60 fps on a decent android phone (one+3). and more than 30 fps on my old nexus 7 (2012). The main advantage of the technique are great looking soft shadows with minimal development effort. The code was written for android and renderscript but the concepts are so simple it'll be easy to port to anything else.Just fake it!
The idea here is that we're not going to get into any complex calculations or mind-bending projection of our game world, we're just going to fake it. The algorithm isn't "pixel perfect", it's essentially a hack and it's probably not the fastest technique but the advantages definitely outweigh the drawbacks for quick and small projects.Base concept
The concept is to paint every shadow-caster in black several times with increasing scale, Apply some blur and you're done. By tweaking the bluring and the scaling steps, you get something like the pictures below.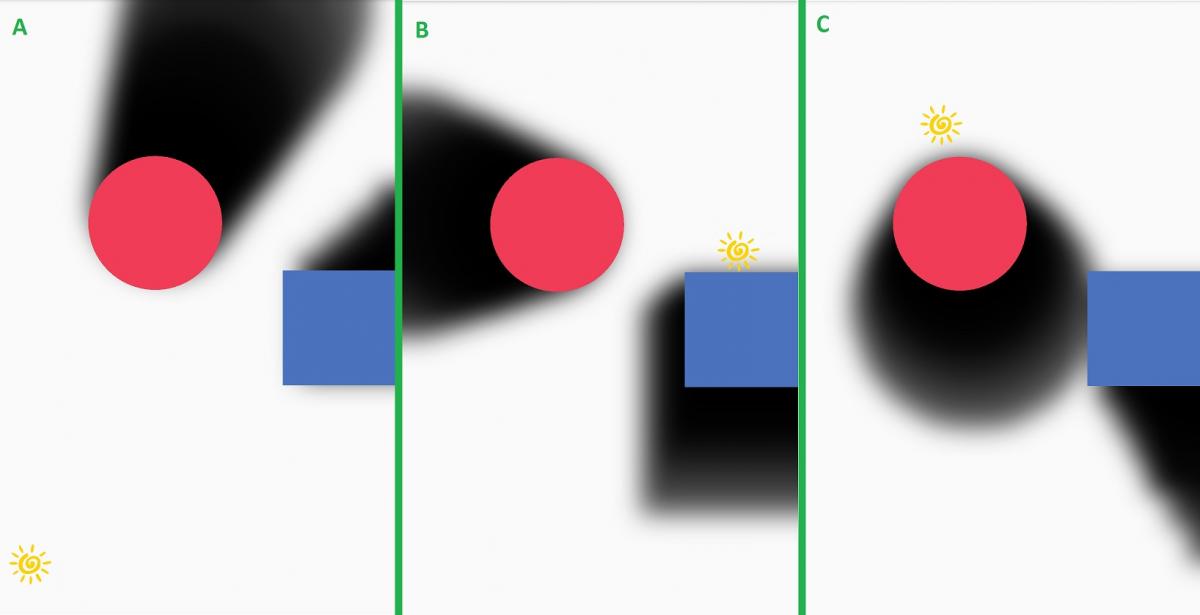
An improved approach
The problem with the algorithm above was that we need multiple render passes for longer shadows, making things really slow, especially on a phone or tablet. The idea is to paint the shadow-casters once in black and then for each pixels, to advance in steps towards the light until a black pixel is encountered. In the picture below, pixel A will be in the shade while pixel B will be in the light.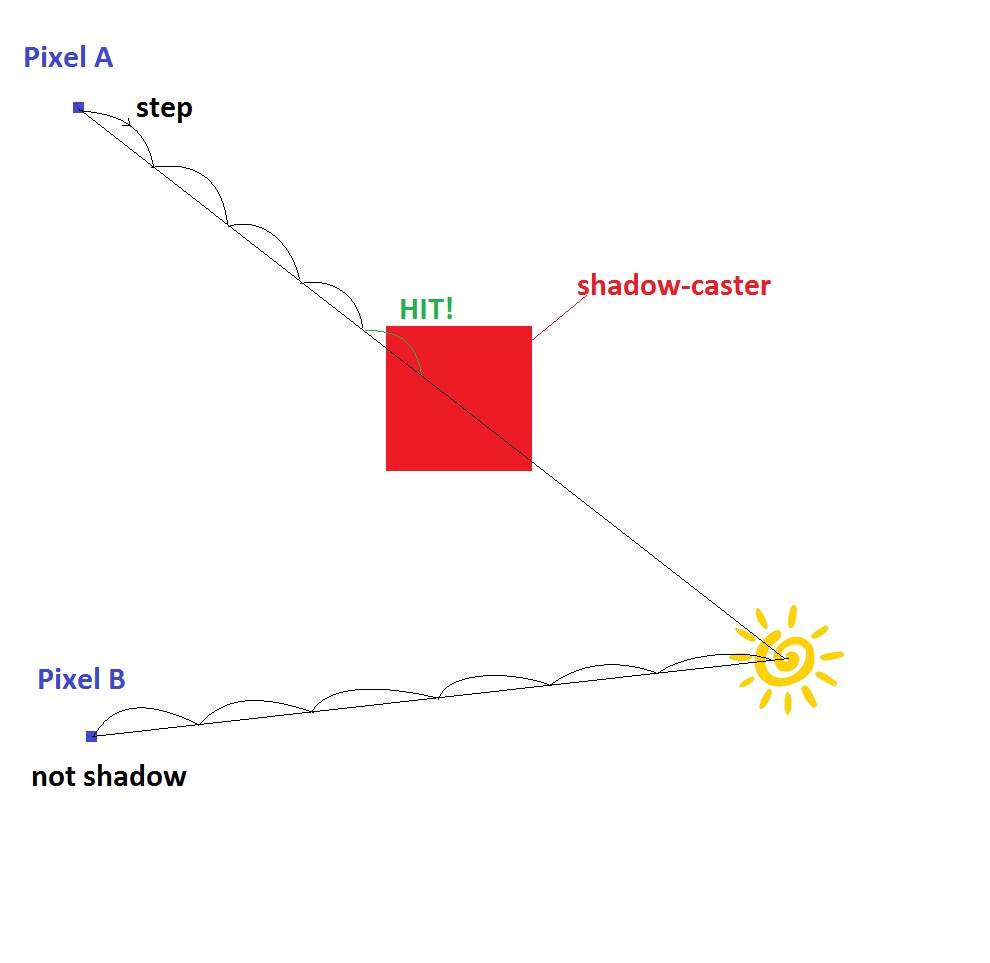
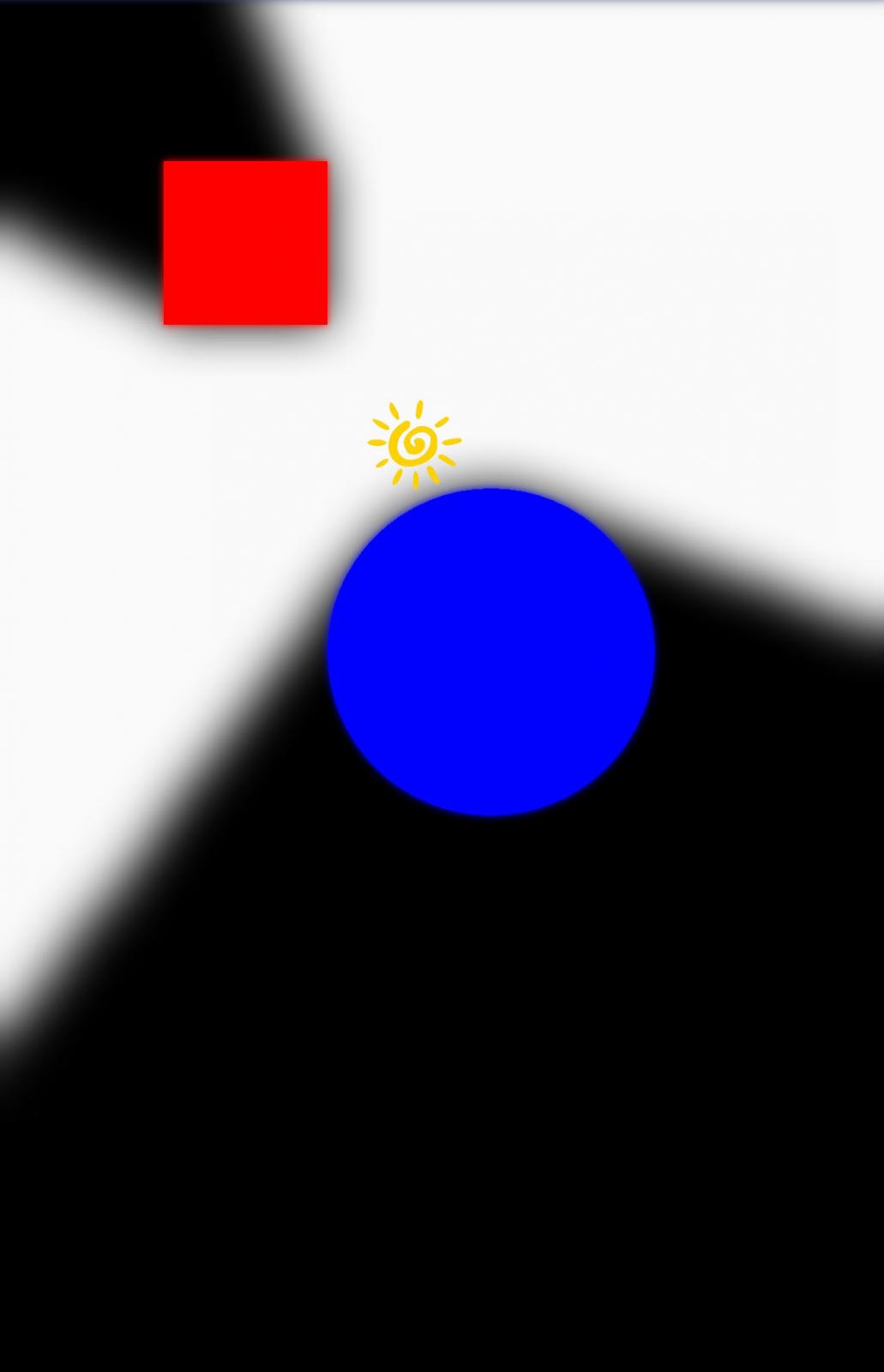
Optimization
The most important optimization to keep in mind is that the shadow computing pass and the blurring pass should always be done on a scaled down image. We can also hardware accelerate the work. The following bit of code is a RenderScript script (Android exclusive) that will run on every pixel on an image where shadow-casters are painted in black, and every other pixel is transparent.
#pragma version(1)
#pragma rs java_package_name(com.olgames.hideandeatbrains.painting)
//these variables are set directly from java code.
float light_x;
float light_y;
float stepSize;
// The input allocation is a copy of the Bitmap, giving us access to all the pixels of the bitmap.
rs_allocation input;
// out contains the currently processed pixel while x and y contain the indices of that pixel.
void root(uchar4 *out, uint32_t x, uint32_t y) {
// First get the current pixel's value in RGBA.
float4 pixel = convert_float4(out[0]).rgba;
// if the current pixel is already drawn to, we can early exit.
if(pixel.a>0.f) {
return;
}
// We'll increment this variable as we go.
float currentStep = stepSize;
// We need to calculate the distance to the light in order not to step past it.
float dX = x-light_x;
float dY = y-light_y;
float dist = sqrt(pow(dX,2)+pow(dY,2));
// we loop and check for "collision" until either we collide or until we step past the light.
// if we collide, we set the current pixel to black.
while(dist>currentStep) {
float4 in = convert_float4(
rsGetElementAt_uchar4(
input,
floor(x-((dX/dist)*currentStep)+0.5f),
floor(y-((dY/dist)*currentStep)+0.5f)
)).rgba;
if (in.a >0.f) {
pixel.r=0;
pixel.g=0;
pixel.b=0;
pixel.a = 255;
out->xyzw = convert_uchar4(pixel);
break;
}
currentStep+= stepSize;
}
}
We'll still need to apply blurring. RenderScript offers very efficient API for that purpose on android.
Conclusion
I almost gave up on implementing shadows for my androidgame because I simply did not have the time to implement more complex algorithms. This technique can be implemented in a few hours. I sincerely hope this will be useful to some of you.Article Update Log
1 Feb 2017: Initial release
"Software engineer in the geo-spatial industry in Belgium."
Just say "Luciad" ;)