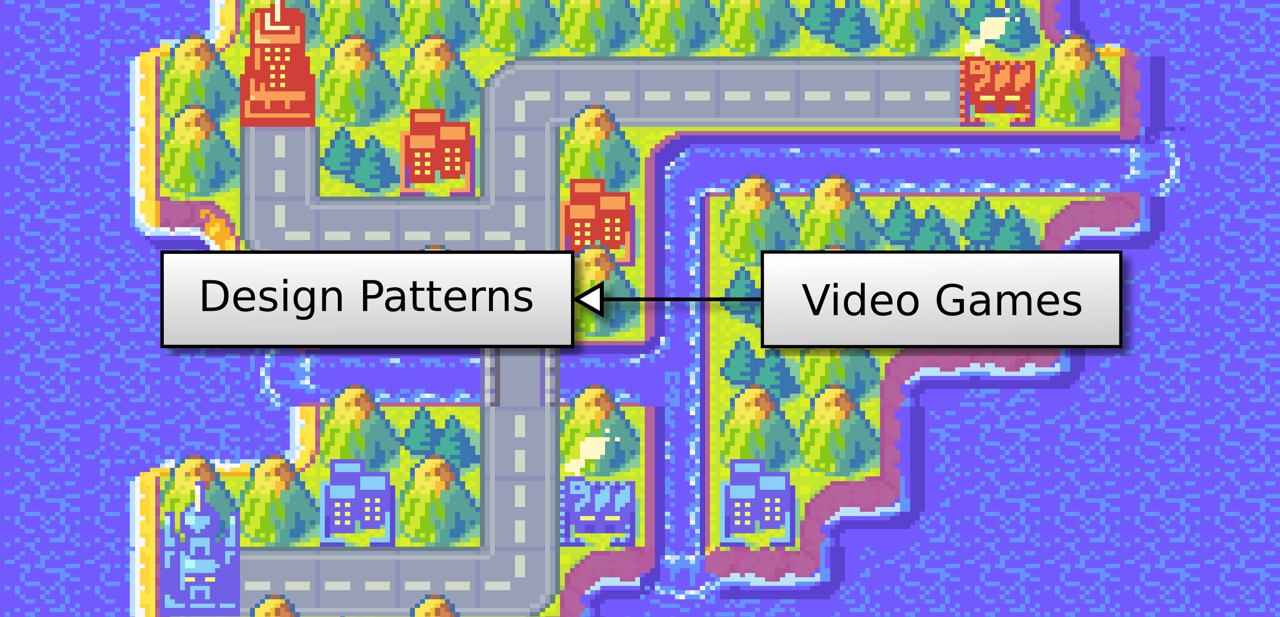
I propose to introduce Python classes to implement the Game Loop pattern. Using these tools, I show how to refactor the code in the previous post to get a more robust and readable program.
This post is part of the Discover Python and Patterns series
Create a class with PythonIn this post, I introduce…
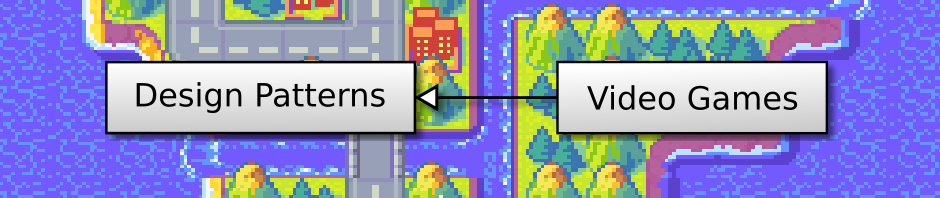
Thanks to the Pygame library we installed in the previous post, we can draw 2D graphics. In this post, I propose to introduce controls with the keyboard as well as some improvements like window centering and frame rate handling.
This post is part of the Discover Python and Patterns series
Keyboard ev…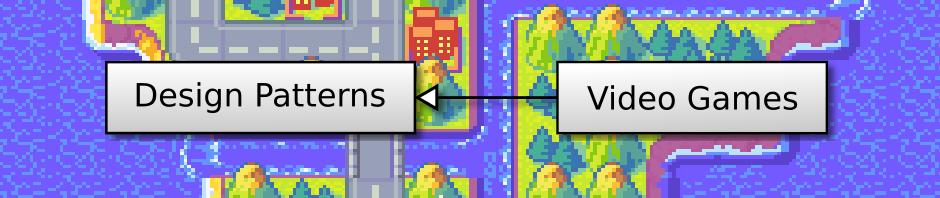
In the previous posts, I introduced enough of Python and patterns to start creating games with graphics using the Pygame library.
This post is part of the Discover Python and Patterns series
Install PygamePygame is a popular library for creating 2D games. Like the random library we used before, the i…
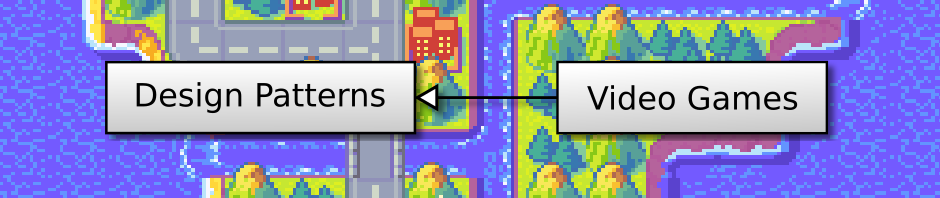
The time has come to see our first design pattern: the Game Loop Pattern! This pattern can give us many good ideas to refactor our game in a very effective way.
This post is part of the Discover Python and Patterns series
The Game Loop patternThere is several version of the Game Loop pattern. Here I …
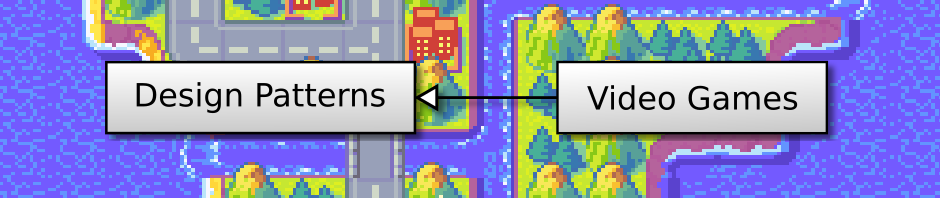
It is now time to organize/refactor our code! When you begin, this is a strange process since the final code does the same as before. However, refactoring is the only way to create a code easy to maintain and expand.
This post is part of the Discover Python and Patterns series
Define a functionIn the…
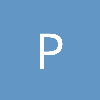
In the previous post, the magic number is always the same. I propose now to introduce random numbers to change the magic number every time we launch the game. I also present imports and more on integers.
This post is part of the Discover Python and Patterns series
Library importIn the previous progra…
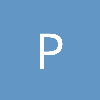
In previous posts, I only used strings and booleans. In this one, I use a new data type called “int” (for integer) that allows the storage and computation of natural numbers (numbers without a decimal part). I use them to create the famous “guess the number” game!
This post is part of the Discover P…
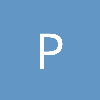
The previous game only allows one try: you have to restart it to propose another word. In this post, I introduce loops, and I use them to repeat the game until the player finds the magic word.
This post is part of the Discover Python and Patterns series
While statementThe while statement allows to re…
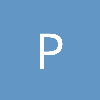
Programs in the previous post always lead to the same result. Here, I show you the basics of branching, or how to change the flow of a program depending on cases.
If statementIf we want to display a message when the player types a specific word, we can use the following syntax:
word = input("Enter th…
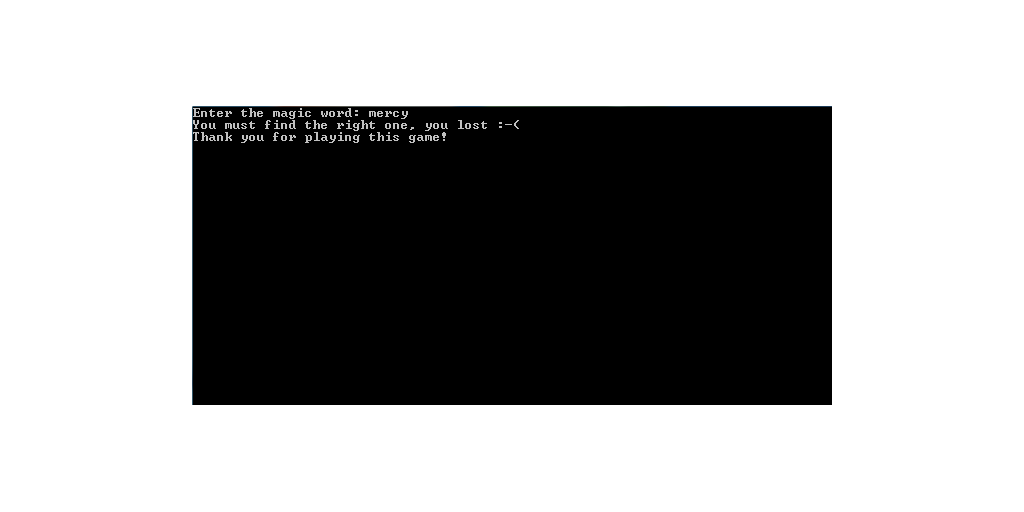
In the first post, we saw how to install an IDE and how to display a message; it is time to add some interaction with the player.
Wait for a keyCreate a new python program and type the following line:
input("Press Enter to continue...")
If you run the program, you get the follo…
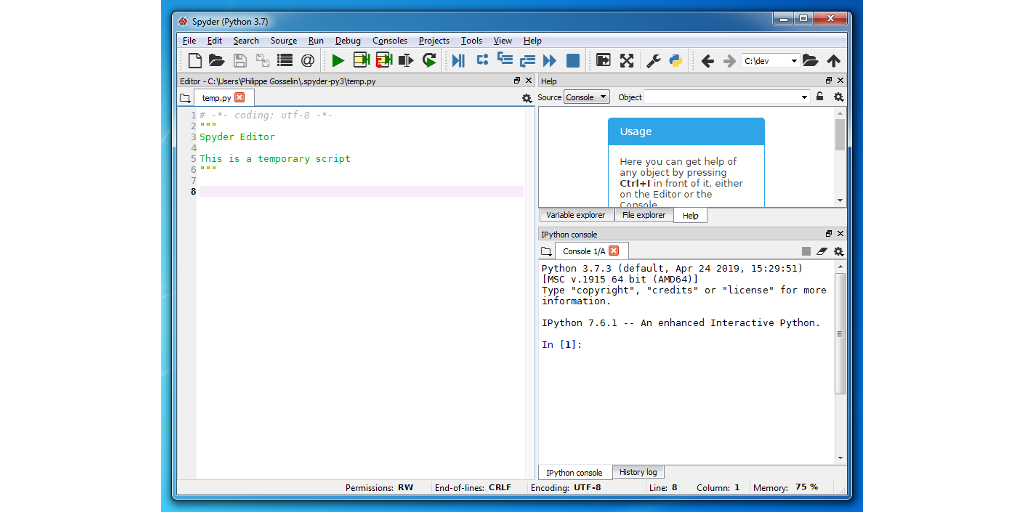
In this series, I propose an introduction to programming for beginners. To do so, I choose the Python language, which is today one of the best (if not the best) language to discover programming. And, as you may guess, we’ll program games
Before starting anything we need a development environ…
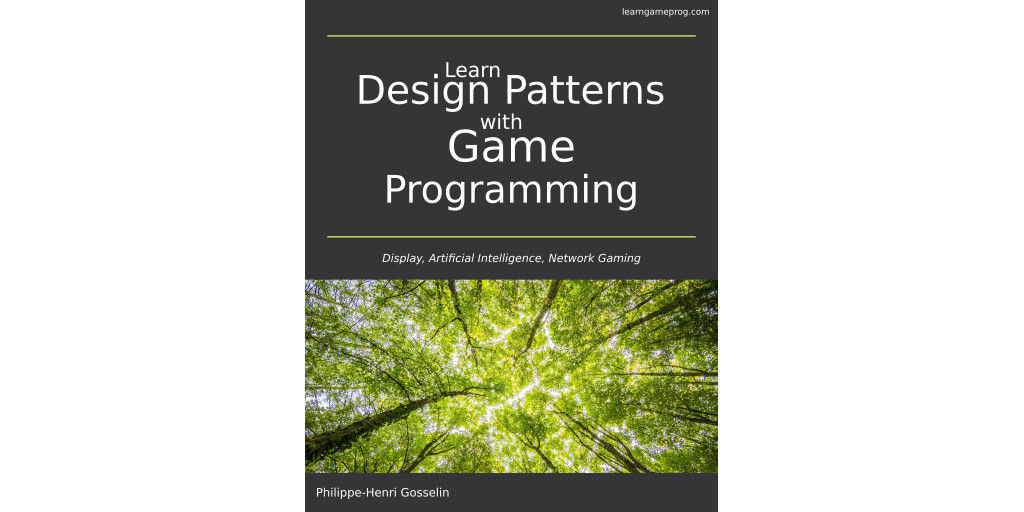
The paperback version of my book "Learn Design Patterns with Game Programming" is finally out!
The Kindle version is updated with some improvements and is still freely available if you subscribed to Kindle Unlimited.
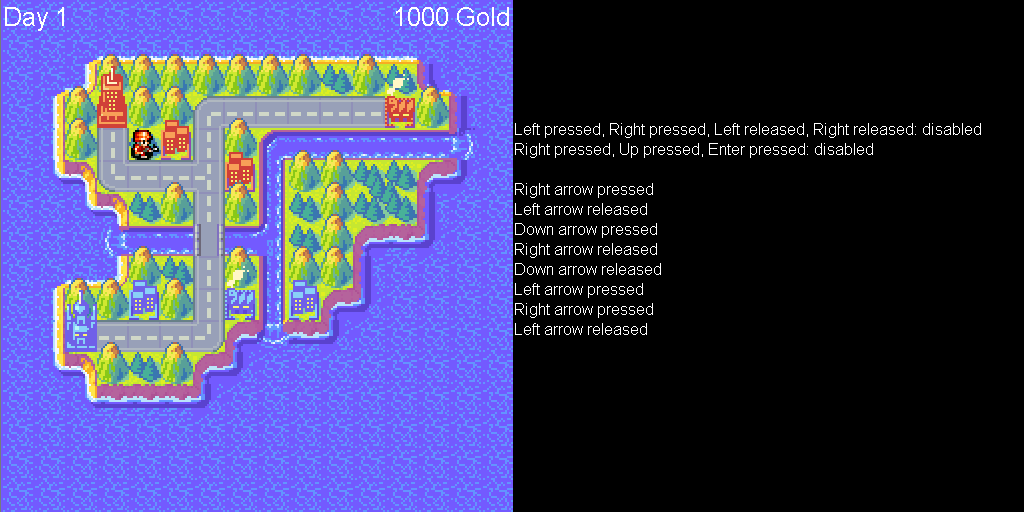
In this post, I add the keyboard management to the facade, and I propose a way to detect key sequences. These sequences are used, for example, in games like Street Fighter II, where characters move according to specific key combos.
Keyboard facadeI first design the keyboard facade. As I…
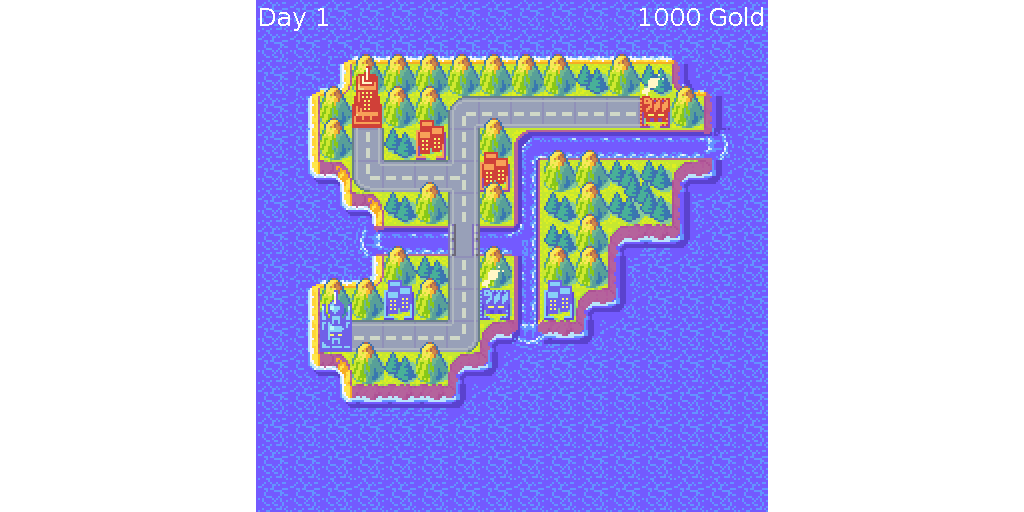
Graphic libraries usually provide methods to draw text on the screen. These handy methods are often quite slow to run because they recompute many parameters at each call. To save computational time, the flyweight pattern can be used to provide text parameters with low memory and cpu usage.
F…
After loading a level, I now propose to add interaction with the use of a mouse. This will be an opportunity to see two other patterns: the Observer Pattern to handle mouse events, and the Game Loop Pattern for synchronization between controls, updates, and display.
This post is part of the…
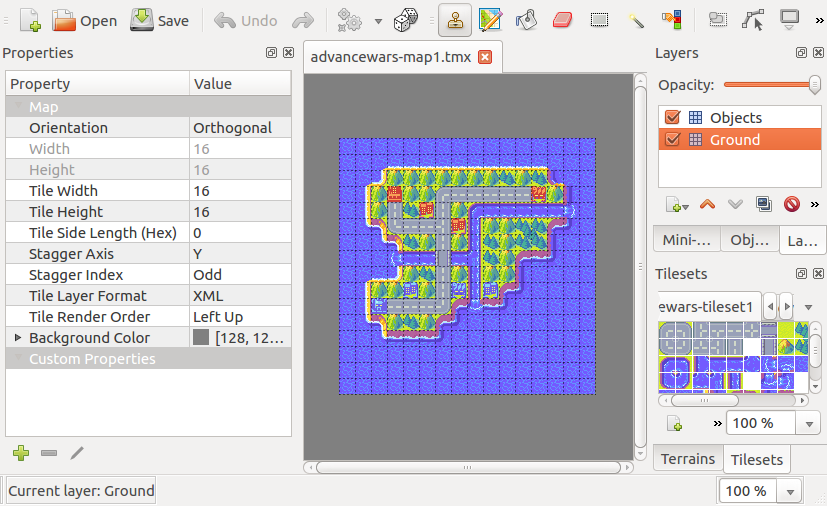
In this article, I propose to use the Visitor Pattern to easily load a level. The GUI facade in the previous post is used to display the level. This pattern allows (among other things) to easily browse a data structure to extract information. In our case, it is an XML file created by “Tiled Map …
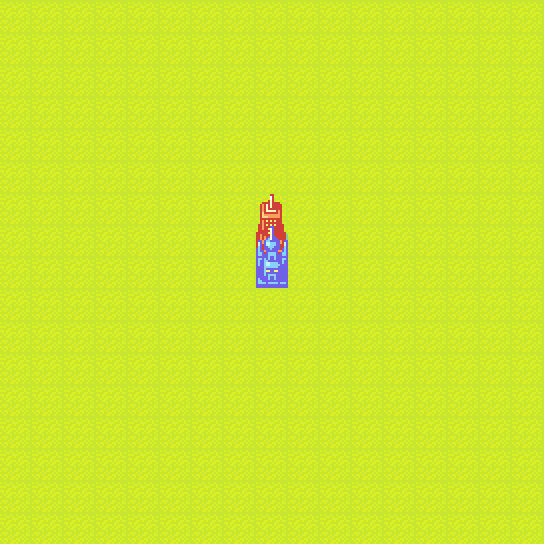
I continue the extension of the graphic facade (see previous article), with here the addition of a very classic form of drawing for video games: tiles. These make it possible to compose an image using small images called tiles. There are several types of tiles, I propose in this article the simp…

The facade seen in the previous article has only an interface with its methods. It is also possible to enrich a facade with additional interfaces, connected to each other by various means. To illustrate it, I propose to add image management. This addition also makes it possible to present anothe…

In this article, I propose to continue the design of a facade for a 2D tile game (previous post). I add two new features: creation/destruction of the window, and basic drawing. Each feature is managed by a batch of methods in a GUIFacade interface.
This post is part of the AWT GUI Facade ser…

In this series, I propose to discover the Facade Pattern. In short, this pattern defines an interface that allows a reduced and simplified use of a set of features. To illustrate, we design a facade of the AWT graphic library for a 2D tile game.
This post is part of the AWT GUI Facade series…