Hello,
I am painting a fully opaque texture and then a semitransparent texture to a DirectX Rendertarget of type R8G8B8A8_UNORM.
However it seems that the alpha channel is not blended correctly even though I am using the blendfunc:
Src: SourceAlpha - Dest: InverseSourceAlpha
It seems as if the texture that gets painted last doesnt even care what is already in the Dest buffer of the RenderTarget. It just overwrites any existing alpha value. Debugging the rendertarget's alphachannel with RenderDoc looks like this:
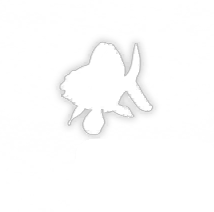
However since the background texture is fully opaque, I expect also the whole Rendertarget Image to be fully opaque. I.e. the alpha channel should be fully white.
If I paint the same textures to the Swapchain Backbuffer the alphachannel gets blended normally.