Step-by-step instruction of Snake 2D using JavaScript/ES5, Canvas API
Let's make a very simple classic snake game. For example, if we have a snake head with 10x10 pixels then we will move our snake by a step with 10 pixels using timer.
This is the result of our work: Sandbox
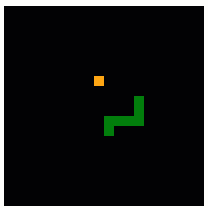
Note. I take ideas from this tutorial: Python Snake Game
We need to get a canvas context from the canvas element. The canvas context is an object that have methods for working with graphics. Lets' create a script element inside of "index.html" file, get the canvas element, get the canvas context and set a clear color to black:
!DOCTYPE html> <head> <title>Snake</title> </head> <body> <canvas width="200" height="200" id="gameCanvas"> Your browser does not support HTML5 Canvas. Please shift to a newer browser. </canvas> <script> var canvas, ctx; canvas = document.getElementById("gameCanvas"); ctx = canvas.getContext("2d"); // Clear the canvas element ctx.clearRect(0, 0, canvas.width, canvas.height); // Set a clear color ctx.fillStyle = "black"; // Fill the canvas element with the clear color ctx.beginPath(); ctx.rect(0, 0, canvas.width, canvas.height); ctx.fill(); </script> </body> </html>
Write a function for drawing a rectangle and call this function for test:
<!DOCTYPE html> <head> <title>Snake</title> </head> <body> <canvas width="200" height="200" id="gameCanvas"> Your browser does not support HTML5 Canvas. Please shift to a newer browser. </canvas> <script> var canvas, ctx; canvas = document.getElementById("gameCanvas"); ctx = canvas.getContext("2d"); // Clear the canvas element ctx.clearRect(0, 0, canvas.width, canvas.height); // Set a clear color ctx.fillStyle = "black"; // Fill the canvas element with the clear color ctx.beginPath(); ctx.rect(0, 0, canvas.width, canvas.height); ctx.fill(); // Call a drawRectangle() function drawRectangle(0, 20, "green", 20); function drawRectangle(x, y, color, size) { ctx.beginPath(); ctx.rect(x, y, size, size); ctx.fillStyle = color; ctx.fill(); } </script> </body> </html>
Each game must have a game loop that will be called by timer. I created the GameLoop method that just prints "Hello, World!" to the debug console every 500 ms:
setInterval(gameLoop, 500) function gameLoop() { console.log("Hello, World!"); }
For a while our GameLoop will have only two called methods Update() and Draw(). The Update() method will have updates for snake cell coordinates and etc. The Draw() method will have only draw methods for game entities.
function gameLoop() { update(); draw(); } function update() { console.log("update"); } function draw() { drawSnake(); drawFood(); } function drawSnake() { console.log("draw snake"); } function drawFood() { console.log("draw food"); }
List data structure is ideal for keeping snake cells coordinates:
// Snake list of (x, y) positions var snake = [{ x: 10, y: 10 }];
Create a function for clearing the canvas:
function draw() { clearCanvas("black") drawSnake(); drawFood(); } function clearCanvas(color) { // Clear the canvas element ctx.clearRect(0, 0, canvas.width, canvas.height); // Set a clear color ctx.fillStyle = color; // Fill the canvas element with the clear color ctx.beginPath(); ctx.rect(0, 0, canvas.width, canvas.height); ctx.fill(); }
Draw the snake:
function drawSnake() { snake.forEach(cell => { drawRectangle(cell.x, cell.y, "green"); }); }
For moving the snake we need to create the "snakeDir" variable:
// Snake movement direction var snakeDir = { x: 10, y: 0 };
The snake moving is very simple. Please, read comments:
function update() { // Calc a new position of the head var newHeadPosition = { x: snake[0].x + snakeDir.x, y: snake[0].y + snakeDir.y } // Insert new position in the beginning of the snake list snake.unshift(newHeadPosition); // Remove the last element snake.pop(); }
I will explain eating food later. But you can read comments in the code.
The result code: Sandbox
<!DOCTYPE html> <head> <title>Snake</title> </head> <body> <canvas width="200" height="200" id="gameCanvas"> Your browser does not support HTML5 Canvas. Please shift to a newer browser. </canvas> <p>Click on this window to activate keyboard handlers to control the snake by WASD and arrow keys.</p> <script> // Canvas element and context var canvas, ctx; // Snake list of (x, y) positions var snake = [{ x: 10, y: 10 }]; // Snake movement direction var snakeDir = { x: 10, y: 0 }; // Snake step var cellSize = 10; // Food var food = { x: 0, y: 0 }; canvas = document.getElementById("gameCanvas"); ctx = canvas.getContext("2d"); // Field size var fieldWidth = canvas.width; var fieldHeight = canvas.height; // Generate an initial random position for the food generateFood(); // Set a key handler document.onkeydown = (event) => { switch (event.keyCode) { case 38: // Up case 87: // W snakeDir.x = 0; snakeDir.y = -cellSize; break; case 37: // Left case 65: // A snakeDir.x = -cellSize; snakeDir.y = 0; break; case 39: // Right case 68: // D snakeDir.x = cellSize; snakeDir.y = 0; break; case 40: // Down case 83: // S snakeDir.x = 0; snakeDir.y = cellSize; break; } }; // Run Game Loop setInterval(gameLoop, 200) function gameLoop() { update(); draw(); } function update() { // Calc a new position of the head var newHeadPosition = { x: snake[0].x + snakeDir.x, y: snake[0].y + snakeDir.y } // Insert new position in the beginning of the snake list snake.unshift(newHeadPosition); // Remove the last element snake.pop(); // Check a collision with the snake and the food if (snake[0].x === food.x && snake[0].y === food.y) { snake.push({ x: food.x, y: food.y }); // Generate a new food position generateFood(); } } function draw() { clearCanvas("black") drawSnake(); drawFood(); } function clearCanvas(color) { // Clear the canvas element ctx.clearRect(0, 0, canvas.width, canvas.height); // Set a clear color ctx.fillStyle = color; // Fill the canvas element with the clear color ctx.beginPath(); ctx.rect(0, 0, canvas.width, canvas.height); ctx.fill(); } function drawSnake() { snake.forEach(cell => { drawRectangle(cell.x, cell.y, "green", cellSize); }); } function drawFood() { drawRectangle(food.x, food.y, "orange", cellSize); } function drawRectangle(x, y, color, size) { ctx.beginPath(); ctx.rect(x, y, size, size); ctx.fillStyle = color; ctx.fill(); } function generateFood() { food.x = 10 * getRandomInt(0, fieldWidth / 10 - 1); food.y = 10 * getRandomInt(0, fieldHeight / 10 - 1); } // Returns a random integer between min (inclusive) and max (inclusive) function getRandomInt(min, max) { min = Math.ceil(min); max = Math.floor(max); return Math.floor(Math.random() * (max - min + 1)) + min; } </script> </body> </html>