Simple, dumb, reflection
Are there any simple methods to manipulate a set of texture coordinates in a vertex shader to give the illusion of reflection on flat surfaces? Simple as in needing nothing but the vertex, view, projection, and/or camera data? Multiple textures and material blending is already available. I just need to come up with some vertex shader code to move one set of texture coordinates about to give the faint illusion of a reflection. Is that possible?
An example of where it would be used is the lens on a pair of eye-glasses, or a flat pane of glass. I don't want or need actual reflections. Even simple glimmers of light would probably suffice.
Cube mapping seems to require six textures and/or special resource management. Other gimmicks I've seen require data to be encoded into the vertices.
Spherical environment mapping is great, but doesn't have a very pleasant effect on rigid or flat vertex normal surfaces (the looked-up texture coordinate stretches all the way across the flat part of the surface). Are there any spins on that algorithm for flat surfaces?
Thanks for any information.
Look up planar reflections on google or gamedev, I think that's what you want. There are lots of articles, but this one gave me all the help I wanted:
http://www.riemers.net/eng/Tutorials/XNA/Csharp/series4.php
It describes how to create a mirror surface (water).
There were some minor errors, but I can't remember where.
I'll give you a small run down of the procedure to render reflections:
1 - Render everything that will be reflected using an inverted camera to a texture.
2 - Find the projected texture coordinates of each vertex.
3 - Render the texture of (1) with those texture coordinates on the reflected surface.
http://www.riemers.net/eng/Tutorials/XNA/Csharp/series4.php
It describes how to create a mirror surface (water).
There were some minor errors, but I can't remember where.
I'll give you a small run down of the procedure to render reflections:
1 - Render everything that will be reflected using an inverted camera to a texture.
2 - Find the projected texture coordinates of each vertex.
3 - Render the texture of (1) with those texture coordinates on the reflected surface.
I appreciate your help, but I don't want to actually reflect anything. I just want to render a second texture at an offset to give a faint appearance of reflection.
This is an example of what I would like to do:
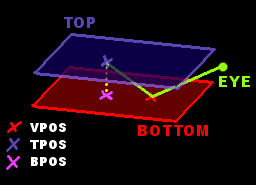
I would like to offset one of the textures applied to BOTTOM in a way that causes the texture coordinate at BPOS to be moved to the location of VPOS when the eye is looking from that perspective.
One simple trick I could do is render a second plane directly under BOTTOM, and make BOTTOM slightly transparent. The second plane would look like a reflection of the ceiling. But rather than do something messy like that, I want to achieve the exact same effect by just moving one of the blended texture coordinates on BOTTOM.
I'm not sure how to go about doing it. I can't seem to come up with vertex shader code that moves the texture correctly for any given surface normal.
This is an example of what I would like to do:
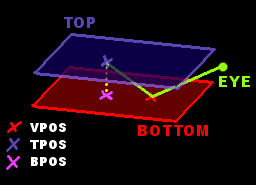
I would like to offset one of the textures applied to BOTTOM in a way that causes the texture coordinate at BPOS to be moved to the location of VPOS when the eye is looking from that perspective.
One simple trick I could do is render a second plane directly under BOTTOM, and make BOTTOM slightly transparent. The second plane would look like a reflection of the ceiling. But rather than do something messy like that, I want to achieve the exact same effect by just moving one of the blended texture coordinates on BOTTOM.
I'm not sure how to go about doing it. I can't seem to come up with vertex shader code that moves the texture correctly for any given surface normal.
Ok, sorry about misunderstanding your question. My best guess would be to fire a ray from the eye to each bottom vertex and reflect it based on the normal. Then do a ray to plane intersection test with a plane placed at the top position in your drawing. This new point will be your texture coordinate (you must of course normalize the texture coord to get it in the range of 0 to 1).
If you do this, I believe that the texture will be correctly rendered.
If i'm wrong, post your vertex shader code so I can take a look.
If you do this, I believe that the texture will be correctly rendered.
If i'm wrong, post your vertex shader code so I can take a look.
Quote:
Ok, sorry about misunderstanding your question. My best guess would be to fire a ray from the eye to each bottom vertex and reflect it based on the normal. Then do a ray to plane intersection test with a plane placed at the top position in your drawing. This new point will be your texture coordinate (you must of course normalize the texture coord to get it in the range of 0 to 1).
If you do this, I believe that the texture will be correctly rendered.
that is correct but you can also do it without the reflection, because as th OP already stated, you get the same effect if you calculate the intersection of the ray and a plane below the surface like this:
___________/____ Top \ / \ /_______\/_____ Bottom / /_____/________ Top'
in pseudocode that means:
Vector3 viewdir;//normalized view direction in tangentspace
float height;//how far the 2 planes should be apart
float t=height/viewdir.y;
Vector2 Offset=Vector2(viewdir.x,viewdir.z)*t; //this is the offset that you have to add to the texture coordinates
regards,
m4gnus
In my proto-engine (www.badfoolprototype) I've developed planar reflection, and actually it works fine!
I can suggest you to look at this article:
http://http.developer.nvidia.com/GPUGems2/gpugems2_chapter19.html
It is about refraction, but the water example has planar reflection.
Download the entire code of the chapter 19 here:
http://http.download.nvidia.com/developer/GPU_Gems_2/CD/Index.html
It is a good way to start, simple and it works!
Hope it helps!
I can suggest you to look at this article:
http://http.developer.nvidia.com/GPUGems2/gpugems2_chapter19.html
It is about refraction, but the water example has planar reflection.
Download the entire code of the chapter 19 here:
http://http.download.nvidia.com/developer/GPU_Gems_2/CD/Index.html
It is a good way to start, simple and it works!
Hope it helps!
JorenJoestar: Thanks for the links. I'll definitely look into that technique.
I can manage the absolutely vertical situation. My trouble is performing the routine for any given surface normal. The problem becomes more complex when the vertex normal dictates the direction of the plane offset. I suspect it may not even be possible without additional information. Namely, a relationship between world space and texture space. In any case, I appreciate the help.
Quote:Original post by m4gnus
in pseudocode that means:
Vector3 viewdir;//normalized view direction in tangentspace
float height;//how far the 2 planes should be apart
float t=height/viewdir.y;
Vector2 Offset=Vector2(viewdir.x,viewdir.z)*t; //this is the offset that you have to add to the texture coordinates
I can manage the absolutely vertical situation. My trouble is performing the routine for any given surface normal. The problem becomes more complex when the vertex normal dictates the direction of the plane offset. I suspect it may not even be possible without additional information. Namely, a relationship between world space and texture space. In any case, I appreciate the help.
This topic is closed to new replies.
Advertisement
Popular Topics
Advertisement