Becomes...
[/quote]
(I used Quote tags so the white in the images doesn't blend with the post background.)
I would change the offset to crop throughout the map as the player moved, which would equal camera scrolling. Can someone give me some example SDL code for doing this? I'm sure it is simple. I just don't understand it. I can already do it with an image and make scrolling backgrounds. It is just making the player and enemies and such move with the camera that is confusing. Please clear this up for me. Thank you.
Screen Cropping SDL
This is one of the few things bugging me. Getting a camera to scroll. I load the whole area, but I need to crop out only a certain part of the screen and display it... like so:
This is usually achieved by managing two co-ordinate spaces. The former image shows "world space co-ordinates", which are offsets from some arbitrary origin (often the top left of the map, but it really doesn't matter). The second image is in "screen space co-ordinates".
When you write your game logic, you keep everything in world space. Then, when you want to draw the next frame, you need to convert the object's world co-ordinates to screen space. Conversely, if a UI event occurs at a particular screen position (typically a mouse click or movement), it needs to be transformed into world space before you do some hit testing.
For small 2D games, the transformation can be as simple as maintaining a camera position (x, y) pair. Each object is drawn at object.x - camera.x, object.y - camera.y. Mouse clicks can be transformed by camera.x - mouse.x, camera.y - mouse.y.
An example of a more complex transformation is to have the world co-ordinates use positive Y as "up" (unlike SDL which uses positive Y as "down"). Another would be to use virtual co-ordinates in world space, ones that do not map directly to pixels. When I write a geometric game, I abstract the world space from integral pixel values to floats in the range 0..1.
When you write your game logic, you keep everything in world space. Then, when you want to draw the next frame, you need to convert the object's world co-ordinates to screen space. Conversely, if a UI event occurs at a particular screen position (typically a mouse click or movement), it needs to be transformed into world space before you do some hit testing.
For small 2D games, the transformation can be as simple as maintaining a camera position (x, y) pair. Each object is drawn at object.x - camera.x, object.y - camera.y. Mouse clicks can be transformed by camera.x - mouse.x, camera.y - mouse.y.
An example of a more complex transformation is to have the world co-ordinates use positive Y as "up" (unlike SDL which uses positive Y as "down"). Another would be to use virtual co-ordinates in world space, ones that do not map directly to pixels. When I write a geometric game, I abstract the world space from integral pixel values to floats in the range 0..1.
So what you are saying is to go through and subtract the camera position from the position of each object on the map, from the player to the blocks that make up the stage itself? This is something that I have tried, but the objects that go under 0, 0 as their coordinates (-100, 0, for example) are rendered at the origin (upper-left-hand corner) of the screen. Perhaps adding if(object[c].x-camera.x<0 || object[c].y-camera.y<0) display[c]=0; (with display[c]=0; disabling displaying the tile) will make the object not be displayed if it has such coordinates? Did I read your response incorrectly, or are we on the same page? Move all of the objects in the map in order to have camera movement? And does the solution I posted work? Thank you for your time. I appreciate it.
EDIT: The problem with the code I posted is that if an object goes beyond 0, 0, it isn't displayed. This is good, but part of the object would be cut off each time. Time for another diagram.
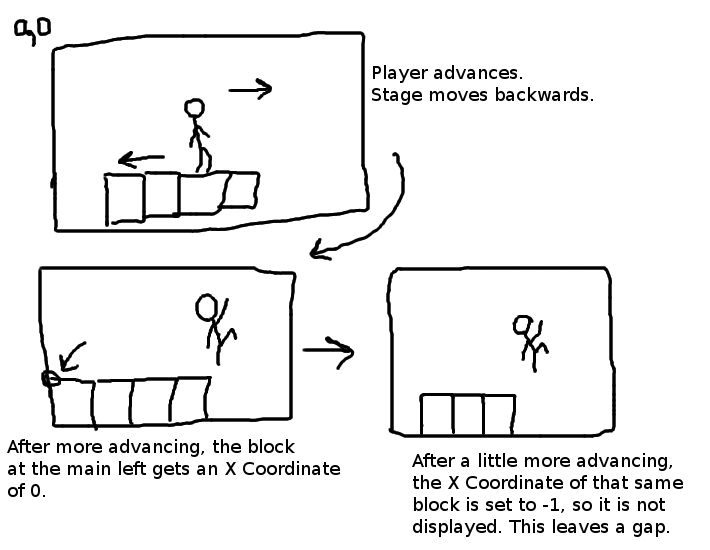
And because the block can't be drawn from before 0 so it is only halfway into or halfway out of the viewing area, that is not an option. How would I avoid such a problem? I know I could put a black border around the screen, but there has to be some other way to do this. Do you understand what I'm saying? Even if the X Coordinate of a block is -400, it is still displayed at 0 on the screen for its X Coordinate.
Again, thanks. I apologize if I am not being easy to understand.
EDIT #2: I made a program prints the X Coordinate of an object after setting that to -400 and it prints 0 instead. I'm going to conclude that object.x and object.y are unsigned integers and therefore can't be set equal to anything less than 0 and be expected to work. All of this is how I got lost in the first place when trying to figure out how to get a camera working. Please give me your insight. Thanks.
EDIT: The problem with the code I posted is that if an object goes beyond 0, 0, it isn't displayed. This is good, but part of the object would be cut off each time. Time for another diagram.
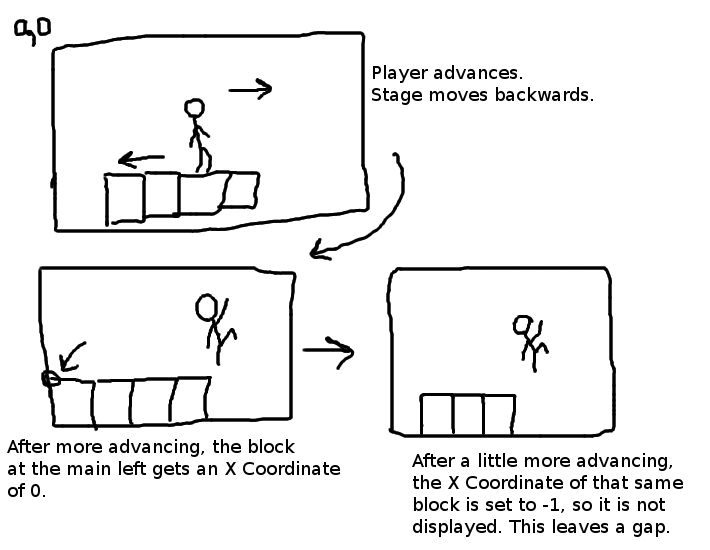
And because the block can't be drawn from before 0 so it is only halfway into or halfway out of the viewing area, that is not an option. How would I avoid such a problem? I know I could put a black border around the screen, but there has to be some other way to do this. Do you understand what I'm saying? Even if the X Coordinate of a block is -400, it is still displayed at 0 on the screen for its X Coordinate.
Again, thanks. I apologize if I am not being easy to understand.
EDIT #2: I made a program prints the X Coordinate of an object after setting that to -400 and it prints 0 instead. I'm going to conclude that object.x and object.y are unsigned integers and therefore can't be set equal to anything less than 0 and be expected to work. All of this is how I got lost in the first place when trying to figure out how to get a camera working. Please give me your insight. Thanks.
You don't move all the objects in the map. Only when you are drawing, do you transform the co-ordinates. Generally speaking, rendering shouldn't modify your objects.
For example:
Forgive my rusty C.
That said, I don't know why negative co-ordinates are causing you trouble. Could you post a minimal example demonstrating this problem?
For example:
struct game_object
{
int x;
int y;
SDL_Surface *sprite;
// ...
};
struct camera
{
int x;
int y;
};
void render(struct game_object *objects, int n, struct camera *camera)
{
// Clear screen
SDL_FillRect(screen, 0, 0);
// Render objects
for(int i = 0 ; i < n ; ++i)
{
struct game_object *object = objects;
int screen_x = object->x - camera.x;
int screen_y = object->y - camera.y;
SDL_Rect dest = { screen_x, screen_y };
SDL_BlitSurface(object->sprite, 0, screen, &dest);
}
// Apply changes
SDL_Flip(screen);
}
int main()
{
// Initialise
int n;
struct game_object *objects = load_game_objects("game_objects.dat", &n);
struct camera camera = { /* ... */ };
int running = 1;
while(running)
{
input(&camera)
game_logic(objects, n);
render(objects, n, &camera);
}
// Cleanup
}
Forgive my rusty C.
That said, I don't know why negative co-ordinates are causing you trouble. Could you post a minimal example demonstrating this problem?
Minimal Example:
I used a 640x480 solid white image for white.png and a 16x16 red dot for dot.png. Substitute these with whatever you have readily available. The player (dot) will not go into negative coordinates no matter what. (Controls are Up, Down, Left, and Right on the keyboard.) I was hoping there would be a way to take the "world space co-ordinates" and crop out the "screen space co-ordinates" without having to shift objects around.
#include <SDL/SDL.h>
#include <SDL/SDL_image.h>
int main() {
SDL_Event kbrd;
SDL_Surface *player, *screen, *background;
player=IMG_Load("dot.png");
background=IMG_Load("white.png");
SDL_Rect playerpos;
playerpos.x=-100;
playerpos.y=-100;
printf("Original Position: %d, %d\n",playerpos.x,playerpos.y);
SDL_Init( SDL_INIT_EVERYTHING );
screen = SDL_SetVideoMode( 640, 480, 32, SDL_SWSURFACE );
unsigned char done=0, keydown=0, direction;
while(!done) {
while ( SDL_PollEvent(&kbrd) ) {
switch (kbrd.type) {
case SDL_KEYDOWN:
#ifndef _WIN32_WCE
if ( kbrd.key.keysym.sym == SDLK_ESCAPE ) {
done = 1;
}
else if(kbrd.key.keysym.sym==SDLK_RIGHT) {
direction=1;
keydown=1;
}
else if(kbrd.key.keysym.sym==SDLK_LEFT) {
direction=2;
keydown=1;
}
else if(kbrd.key.keysym.sym==SDLK_UP) {
direction=3;
keydown=1;
}
else if(kbrd.key.keysym.sym==SDLK_DOWN) {
direction=4;
keydown=1;
}
#else
done = 1;
#endif
break;
case SDL_KEYUP:
if(kbrd.key.keysym.sym==SDLK_RIGHT || kbrd.key.keysym.sym==SDLK_LEFT || kbrd.key.keysym.sym==SDLK_UP || kbrd.key.keysym.sym==SDLK_DOWN) keydown=0;
break;
case SDL_QUIT:
done = 1;
break;
default:
break;
}
}
SDL_BlitSurface(background,NULL,screen,NULL);
SDL_BlitSurface(player,NULL,screen,&playerpos);
SDL_Flip(screen);
if(keydown && direction==1) playerpos.x+=4;
if(keydown && direction==2) playerpos.x-=4;
if(keydown && direction==3) playerpos.y-=4;
if(keydown && direction==4) playerpos.y+=4;
if(keydown) printf("%d, %d\n",playerpos.x,playerpos.y);
}
return(0); }
I used a 640x480 solid white image for white.png and a 16x16 red dot for dot.png. Substitute these with whatever you have readily available. The player (dot) will not go into negative coordinates no matter what. (Controls are Up, Down, Left, and Right on the keyboard.) I was hoping there would be a way to take the "world space co-ordinates" and crop out the "screen space co-ordinates" without having to shift objects around.
The problem is that you are re-using the SDL_Rect you pass to SDL_BlitSurface. SDL_BlitSurface modifies the input rectangle to the clipped surface. You need to generate a copy of the rectangle:
See the SDL documentation for SDL_BlitSurface:
SDL_Rect dest = playerpos;
SDL_BlitSurface(player,NULL,screen,&dest);
See the SDL documentation for SDL_BlitSurface:
[color=#000000][font=Arial,]The final blit rectangle is saved in [/font]dstrect[color=#000000][font=Arial,] after all clipping is performed ([/font]srcrect[color=#000000][font=Arial,] is not modified).[/font]
[/quote]
Ahh! Thanks for clearing that up. It really helped out. This should be all that I need in order to get scrolling working and everything, so thank you very much!
Selecting the green arrow next to "Was this post useful to you?" increases your Reputation, right? I'll be doing that now on all of your posts here. Thanks again!


This topic is closed to new replies.
Advertisement
Popular Topics
Advertisement