I'm going to show you the tests I run now that I know more stuff about textures.
Notice that I'm uploading 5 Textures but using (binding) only the first one (a.jpg)!
Divisible by 4 is yes if (width * channels) / 4.0f is an integer number.
Texture Max Size is the return value of glGetIntegerv(GL_MAX_TEXTURE_SIZE, &size)
Test 1 (Without forcing 4 channels.)
If you see the log, all files are divisible by 4 ( (width * height) / 4) so the alignment is not a problem.
Test 2 (Without forcing 4 channels and Uploading only one texture)
I'm getting what I expected. This seems so weird. How can the upload of the other textures in the first test can impact to that weird result since I'm not even using them?
Test 3 (Forcing 4 channels):
As you can see by forcing 4 channels I'm getting what I expected.
Code:
Notice that in the first and second test stbi_load(path.c_str(), &m_width, &m_height, &m_channels, 0); and also the line m_channels = 4; does not exist.
//Reverse the pixels.
stbi_set_flip_vertically_on_load(1);
//Try to load the image.
//FORCE 4 CHANNELS.
unsigned char *data = stbi_load(path.c_str(), &m_width, &m_height, &m_channels, 4);
m_channels = 4;
//Debug.
float check = (m_width * m_channels) / 4.0f;
printf("file: %20s \tchannels: %d, Divisible by 4: %s, width: %d, height: %d, widthXheight: %d\n",
path.c_str(), m_channels, check == ceilf(check) ? "yes" : "no", m_width, m_height, m_width * m_height);
//Image loaded successfully.
if (data)
{
//Generate the texture and bind it.
GLCall(glGenTextures(1, &m_id));
GLCall(glActiveTexture(GL_TEXTURE0 + unit));
GLCall(glBindTexture(GL_TEXTURE_2D, m_id));
//Not Transparent texture.
if (m_channels == 3)
{
GLCall(glTexImage2D(GL_TEXTURE_2D, 0, GL_RGB, m_width, m_height, 0, GL_RGB, GL_UNSIGNED_BYTE, data));
}
//Transparent texture.
else if (m_channels == 4)
{
GLCall(glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA, m_width, m_height, 0, GL_RGBA, GL_UNSIGNED_BYTE, data));
}
else
{
throw EngineError("UNSUPPORTEWD");
}
//Texture Filters.
GLCall(glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_REPEAT));
GLCall(glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_REPEAT));
GLCall(glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_NEAREST_MIPMAP_NEAREST));
GLCall(glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR));
//Generate mipmaps.
GLCall(glGenerateMipmap(GL_TEXTURE_2D));
}
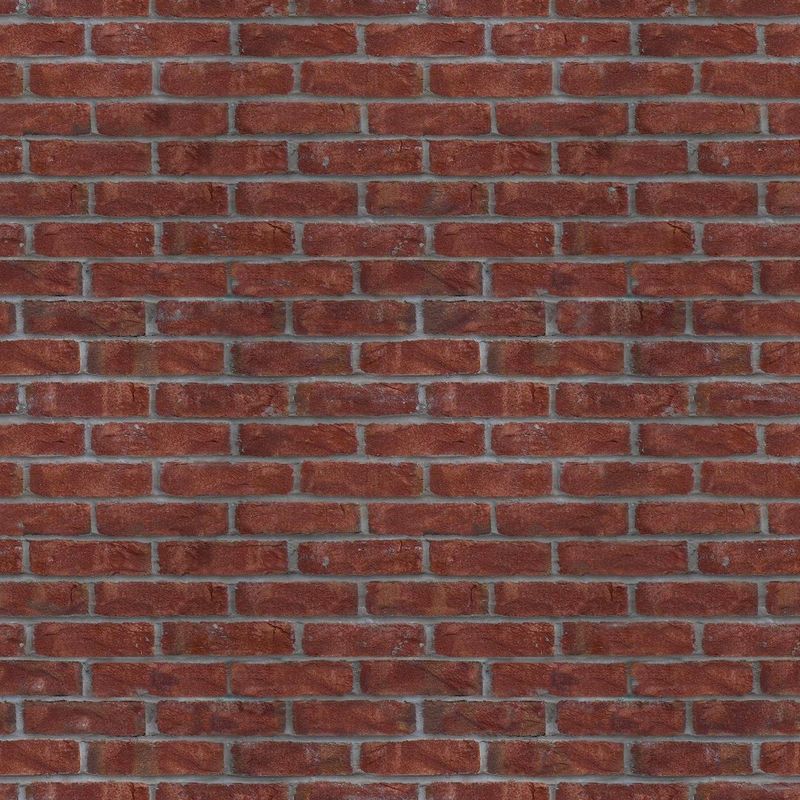
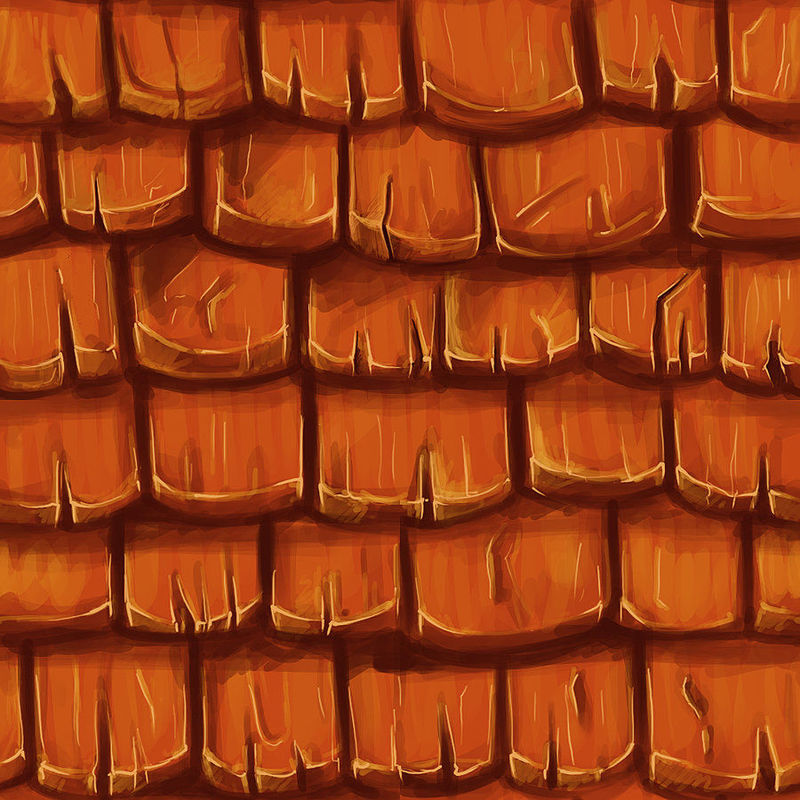
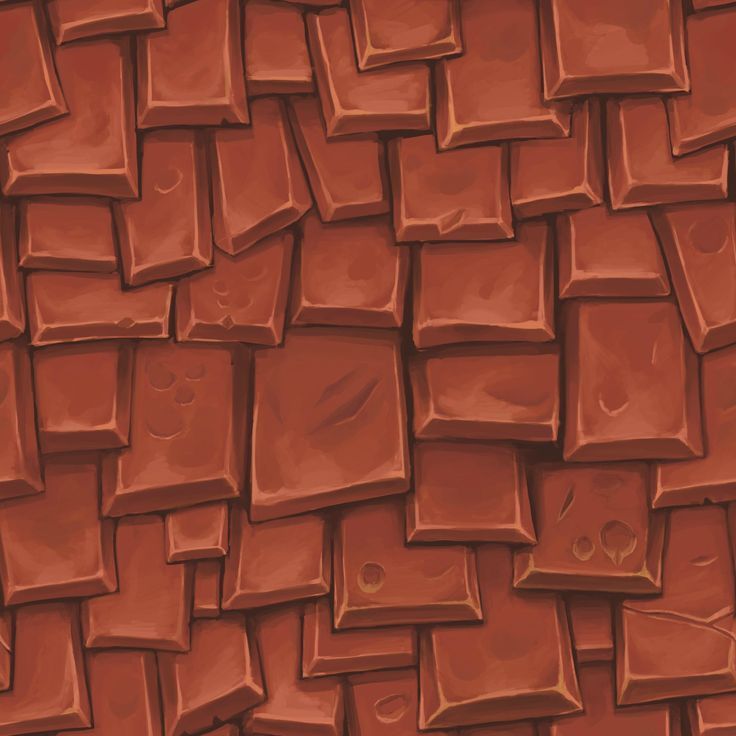
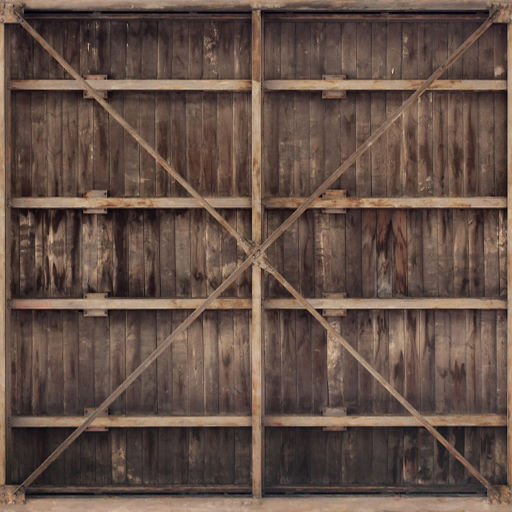
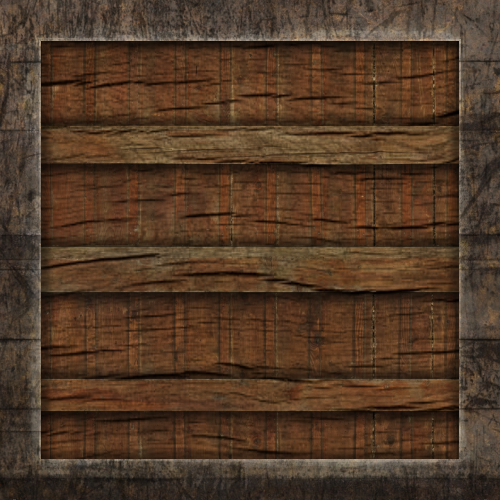