Hello !
I'm totally new to your community and happy to join !
I have a rendering problem since long time and I don't know how to solve it. I develop roguelike game where I display a 2D TopView map based on a simple png file like this :

But, with different game lib, I have the same problem (it seems to be not on all computer) when a resize the windows. A black border is sometime visible (depending windows size).
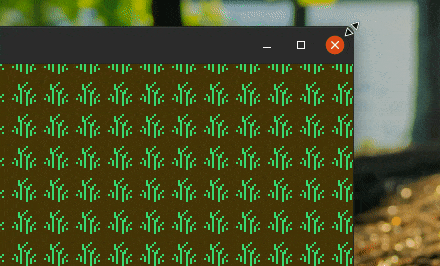
I think it is “float” related. Tiles position, size, etc. are floats. Maybe float usage by gpu make pixel selection “error” on source texture ? I don't know. Any idea about what happens ? And how to solve ?
If you want to try it, the code is in Rust:
Cargo.toml :
[package]
name = "demo_squads"
version = "0.1.0"
edition = "2021"
[dependencies]
macroquad = "0.3"
src/main.rs :
use macroquad::prelude::*;
#[macroquad::main("test")]
async fn main() {
let tile_size = 32.;
let world_size_tiles = 64; // square world
let world_size = world_size_tiles as f32 * tile_size;
let texture = load_texture("test.png").await.unwrap();
let background_tile_source = Rect::new(0., 0., tile_size, tile_size);
let foreground_tile_source = Rect::new(32., 32., tile_size, tile_size);
loop {
clear_background(BLACK);
let zoom_x = (world_size / screen_width()) * 2.;
let zoom_y = (world_size / screen_height()) * 2.;
set_camera(&Camera2D {
zoom: Vec2::new(zoom_x, zoom_y),
offset: Vec2::new(-0.5, 0.),
..Default::default()
});
for row_i in 0..world_size_tiles {
for col_i in 0..world_size_tiles {
let real_dest_x = col_i as f32 * tile_size;
let real_dest_y = row_i as f32 * tile_size;
let camera_dest_x = real_dest_x / world_size;
let camera_dest_y = real_dest_y / world_size;
let dest_size_x = tile_size / world_size;
let dest_size_y = tile_size / world_size;
// background
draw_texture_ex(
texture,
camera_dest_x,
camera_dest_y,
WHITE,
DrawTextureParams {
source: Some(background_tile_source),
dest_size: Some(Vec2::new(dest_size_x, dest_size_y)),
flip_y: true,
..Default::default()
},
);
// foreground
draw_texture_ex(
texture,
camera_dest_x,
camera_dest_y,
WHITE,
DrawTextureParams {
source: Some(foreground_tile_source),
dest_size: Some(Vec2::new(dest_size_x, dest_size_y)),
flip_y: true,
..Default::default()
},
);
}
}
next_frame().await
}
}
And Thanks for your time if you read me !