Quote:
Native Client is an open-source research technology for running x86 native code in web applications, with the goal of maintaining the browser neutrality, OS portability, and safety that people expect from web apps. We've released this project at an early, research stage to get feedback from the security and broader open-source communities. We believe that Native Client technology will someday help web developers to create richer and more dynamic browser-based applications.
http://code.google.com/p/nativeclient/
1) Download and Install the following [ Unless you dont need to ]
Native client,Python 2.4
2) Setup environment variables
PATH: Add your python directory to the path environment variable. For example: C:\python24
NATIVE_CLIENT: the base directory you installed the native client. For example: C:\NativeClient
3) Python Test: The result should be a picture of the earth
->Open up the command prompt
->exectue the command cd %NATIVE_CLIENT%\nacl\googleclient\native_client\tests\earth
->python run.py
4) Install and use the plug-in
cd %NATIVE_CLIENT%/nacl/googleclient/native_client
Ensure to add the extra arguments... --prebuilt firefox_install, otherwise it wont work!
scons.bat --prebuilt firefox_install
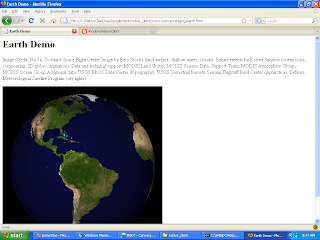
Played around with Googles Native client... allowing you to run x86 C++ code inside the browser. I tried to get to use SDL but didnt manage to, but you can play around with the pixels directly (basing it off the "life sample")

#include #include #if defined(HAVE_THREADS)#include #include #endif#include //#include #include #include #if !defined(STANDALONE)#include #include #else#include "native_client/common/standalone.h"#endiftypedef unsigned int u32;// global properties used to setup lifeconst int kMaxWindow = 4096;const int kMaxFrames = 10000000;int g_window_width = 512;int g_window_height = 512;int g_num_frames = 50000;// seed for rand_r() - we only call rand_r from main thread.static unsigned int gSeed = 0xC0DE533D;// random number helper// binary rand() returns 0 or 1inline unsigned char brand() { return static_cast(rand_r(&gSeed) & 1);}// build a packed colorinline uint32_t MakeRGBA(uint32_t r, uint32_t g, uint32_t b, uint32_t a) { return (((a) << 24) | ((r) << 16) | ((g) << 8) | (b));}struct Color{ u32 argb; Color() { argb=0; } Color(u32 r, u32 g, u32 b, u32 a) { argb = MakeRGBA(r,g,b,a); }};struct Surface{ int w; int h; int d; u32* pixels; Surface(int tw, int th) { w=tw; h=th; d=tw; pixels = new u32[w * h]; for(int i=0; i { pixels= MakeRGBA(0xff,128,0,0); } } virtual ~Surface() { delete [] pixels; } virtual void drawPixel(int tw, int th, u32 argb) { pixels[th * w + tw] = argb; }};struct Box{ int x; int y; int w; int h; u32 color; Box() { } Box(int tx, int ty, int tw, int th, u32 tc) { set(tx,ty,tw,th,tc); } void set(int tx, int ty, int tw, int th, u32 tc) { x=tx; y=ty; h=th; w=tw; color = tc; } void render(Surface* surface) { for(int i=0; i { for(int j=0; j { int tx = x + j; int ty = y + i; surface->drawPixel( tx, ty, color); } } }};struct Canvas { Surface* surface; char *cell_in_; char *cell_out_; Color color; Box b; Box b2; Canvas(Surface* s, const Color& tc) { surface = s; color = tc; cell_in_ = new char[surface->w * surface->h]; cell_out_ = new char[surface->w * surface->h]; for (int i = 0; i < surface->w * surface->h; ++i) { cell_in_ = cell_out_ = 0; } b.set(0,0,100,100, MakeRGBA(0xff,0xff,0xff,0xff)); b2.set(150,150,100,100, MakeRGBA(0xff,0,0x0,0xff)); } virtual ~Canvas() { } virtual void draw() { nacl_video_update(surface->pixels); } virtual void update() { for(int i=0; i< surface->w * surface->h; ++i) { surface->pixels = MakeRGBA(0xff,128,0,0); } b.render(surface); b2.render(surface); } virtual void swapBuffers() { char *temp = cell_in_; cell_in_ = cell_out_; cell_out_ = temp; } virtual bool pollEvents() { NaClMultimediaEvent event; while (0 == nacl_video_poll_event(&event)) { if (event.type == NACL_EVENT_QUIT) { return false; } } return true; }};int main(){ srpc_init(); //attempt to initalize the thing int r = nacl_multimedia_init(NACL_SUBSYSTEM_VIDEO | NACL_SUBSYSTEM_EMBED); if (r == -1 ) { printf("Multimedia system failed to initialize! errno: %d\n", errno); exit(-1); } //attempt to initalize the other thing r = nacl_video_init(NACL_VIDEO_FORMAT_BGRA, g_window_width, g_window_height); if (-1 == r) { printf("Video subsystem failed to initialize! errno; %d\n", errno); exit(-1); } //create our surface Surface *surface = new Surface(g_window_width, g_window_height); Canvas canvas(surface,Color(1,0,0,0)); for (int i = 0; i < g_num_frames; ++i) { canvas.update(); canvas.draw(); canvas.swapBuffers(); if (!canvas.pollEvents()) { break; } }}
Also wrote a simple bitmap reader but I couldnt get fopen to read the damm files.
#pragma pack(1)struct RGBColor{ char r; char g; char b;};struct BitmapHeader{ //must always be set to 'BM' to declare that this is a .bmp-file. short bfType; //specifies the size of the file in bytes. int fileSize; //must always be set to zero. short bfReserved1; //must always be set to zero. short bfReserved2; //specifies the offset from the beginning of the file to the bitmap data. int bfOffBits;};struct BitmapInfo{ int biSize; int biWidth; int biHeight; short biPlanes; short biBitCount; int biCompression; u32 biSizeImage; u32 biXPelsPerMeter; u32 biYPelsPerMeter; u32 biClrUsed; u32 biClrImportant;};#pragma pack()struct Bitmap{ BitmapHeader header; BitmapInfo info; u32* quads; int width; int height; RGBColor* buffer; Bitmap() { width=0; height=0; } Bitmap(const char* fn) { width=0; height=0; load(fn); } int load(const char* fn) { int rc = -1; char tmp[256]; memset(tmp,0,256); memcpy(tmp,fn,strlen(fn)-4); strcat(tmp,"~.bmp"); FILE *f = fopen("testdata256", "wb"); if (NULL != f) { rc=1; } FILE* fp = fopen(fn,"rb"); if(fp) { rc = 1; fseek(fp,0,SEEK_END); //printf("okay\n",fn); u32 fileSize = ftell(fp); u32 headerSize = sizeof(BitmapHeader) + sizeof(BitmapInfo); if(fileSize > headerSize) { rewind(fp); //printf("okay2\n",fn); fread(&header,sizeof(BitmapHeader),1,fp); fread(&info,sizeof(BitmapInfo),1,fp); //printf("header.fileSize:%d\n",header.fileSize); //printf("header.bfReserved1:%d\n",header.bfReserved1); //printf("header.bfReserved2:%d\n",header.bfReserved2); //printf("info.biSize:%d\n",info.biSize); if(header.bfType == 19778 && header.bfReserved1==0 && header.bfReserved2==0 && info.biSize == 40 && info.biBitCount == 24 && info.biCompression == 0) { fileSize = ftell(fp); printf("bfOffBits:%d,fTell:%d\n",header.bfOffBits,fileSize); width = info.biWidth; height = info.biHeight; //printf("width: %d, height:%d, bitsPerPixel:%d, biCompression:%d\n", // width,height,info.biBitCount,info.biCompression); //printf("sizeof color:%d\n",sizeof(Color)); int dataSize = width * height; if(width > 0 && height > 0 && width < 4096 && height < 4096) { buffer = new RGBColor[ dataSize ]; if(buffer) { fread(buffer, dataSize,1,fp); } } //int index = 0; //for(int i=0; i //{ // for(int j=0; j // { // RGBColor c; // memcpy(&c,buffer+index,sizeof(RGBColor)); // //fread(&c,3,1,fp); // if(c.r==0 && c.g==0 && c.b==0) // { // printf("_"); // }else{ // printf("#"); // } // //if(c.r==0) // //{ // // c.r=255; // //} // //if(c.g==0) // //{ // // c.g=255; // //} // //if(c.b==0) // //{ // // c.b=255; // //} // memcpy(buffer+index,&c,sizeof(RGBColor)); // index += sizeof(RGBColor); // } // printf("\t%d\n",i); //} //printf("tmp:%s\n",tmp); //FILE* fp2 = fopen( tmp, "wb"); //if(fp2) //{ // fwrite( &header,sizeof(BitmapHeader),1,fp2); // fwrite( &info,sizeof(BitmapInfo),1,fp2); // fwrite( buffer,dataSize,1,fp2); // fclose(fp2); //} } } } return rc; }};