I also have a resize bug, I think. I've been trying to work around it using a std::mutex. We'll see. because it seems like it bugs out randomly, basically.
Reading ray tracing result to the CPU and back onto the GPU in order to do image denoising
I remember, when working on mobile games, handling those interruptions from the OS side (incoming call, switching to background and back, etc.) was the thing which caused me most paranoia.
Resizing, minimizing etc. is the same kind of problem. In theory you might even loose the GPU context, and then you would need to reupload everything, like if the application has just started up.
Not sure if this can still happen on modern OSes.
That's really bad, frustrating and boring stuff. I never try to fix related bugs at all, if i don't have to. : )
On second thought, I'm going to try that DOF using the path tracer, not the blurring of a bitmap. as a post-process.
taby said:
On second thought, I'm going to try that DOF using the path tracer, not the blurring of a bitmap. as a post-process.
That's the proper attitude. : )
It will give you reference images and the proper math, so the bitmap hack should end up better too.
OK, I'm having some grief. Any ideas? Once I have the get focal point code done, then I'm set.
void main()
{
...
vec4 origin;
vec4 target;
vec4 direction;
get_origin_target_and_direction(
origin,
target,
direction,
gl_LaunchIDEXT.x,
gl_LaunchIDEXT.y,
gl_LaunchSizeEXT.x,
gl_LaunchSizeEXT.y);
float focal_distance = 1.0;
mat4 v;
v = ubo.viewInverse;
vec4 d;
d.x = v[2][0] + ubo.camera_pos.x;
d.y = v[2][1] + ubo.camera_pos.y;
d.z = v[2][2] + ubo.camera_pos.z;
d.w = 0.0;
vec4 focal_point = d * focal_distance;
//direction = focal_point - origin; // no good
...
The code is now:
void main()
{
// Only seed once per pixel
prng_state = gl_LaunchSizeEXT.x * gl_LaunchIDEXT.y + gl_LaunchIDEXT.x;
vec4 origin;
vec4 direction;
get_origin_and_direction(
origin,
direction,
gl_LaunchIDEXT.x,
gl_LaunchIDEXT.y,
gl_LaunchSizeEXT.x,
gl_LaunchSizeEXT.y);
float focal_distance = 1.0;
vec4 focal_point_bootstrap = origin + normalize(direction) * focal_distance;
vec4 focal_point_on_plane = vec4(plane_ray_intersection(focal_point_bootstrap.xyz, (origin - focal_point_bootstrap).xyz, origin.xyz, direction.xyz), 0.0);
// test
//direction = focal_point_on_plane - origin;
//origin = focal_point_on_plane;
// Do chromatic aberration (good for making rainbows via prisms)
const int channels = 3;
//const float max_hue = rgb2hsv(vec3(1.0, 0.0, 1.0)).x; // violet
const float max_hue = rgb2hsv(vec3(0.0, 0.0, 1.0)).x; // blue
const float min_hue = rgb2hsv(vec3(1.0, 0.0, 0.0)).x; // red
const float max_eta = 0.95;
const float min_eta = 0.75;
const float hue_diff = max_hue - min_hue;
const float hue_step_size = hue_diff / (channels - 1);
const float eta_diff = max_eta - min_eta;
const float eta_step_size = eta_diff / (channels - 1);
vec3 grand_color = vec3(0.0);
const int num_samples = 8;
for(int s = 0; s < num_samples; s++)
{
float curr_hue = min_hue;
float curr_eta = min_eta;
vec3 color = vec3(0.0);
// randomize start location
for(int c = 0; c < channels; c++, curr_hue += hue_step_size, curr_eta += eta_step_size)
{
// randomize this
const float f = get_ray0(origin, direction, curr_hue, curr_eta);
const vec3 mask = hsv2rgb(vec3(curr_hue, 1.0, 1.0));
color += f*mask;
}
grand_color += color;
}
grand_color /= num_samples;
//grand_color = pow(grand_color, vec3(2.2));
imageStore(color_image, ivec2(gl_LaunchIDEXT.xy), vec4(grand_color, 1.0));
}
Seems to work good now. Should I be using the bootstrap focal point, or the plane focal point?
Now to randomize some stuff.
It's sort of working:
void main()
{
// Only seed once per pixel
prng_state = gl_LaunchIDEXT.y*gl_LaunchSizeEXT.x + gl_LaunchIDEXT.x;
vec4 origin;
vec4 direction;
get_origin_and_direction(
origin,
direction,
gl_LaunchIDEXT.x,
gl_LaunchIDEXT.y,
gl_LaunchSizeEXT.x,
gl_LaunchSizeEXT.y);
float focal_distance = 1.0;
vec4 focal_point_bootstrap = origin + normalize(direction) * focal_distance;
vec4 focal_point_on_plane = vec4(plane_ray_intersection(focal_point_bootstrap.xyz, (origin - focal_point_bootstrap).xyz, origin.xyz, direction.xyz), 0.0);
// test
//direction = focal_point_on_plane - origin;
//origin = focal_point_on_plane;
// Do chromatic aberration (good for making rainbows via prisms)
const int channels = 3;
//const float max_hue = rgb2hsv(vec3(1.0, 0.0, 1.0)).x; // violet
const float max_hue = rgb2hsv(vec3(0.0, 0.0, 1.0)).x; // blue
const float min_hue = rgb2hsv(vec3(1.0, 0.0, 0.0)).x; // red
const float max_eta = 0.95;
const float min_eta = 0.75;
const float hue_diff = max_hue - min_hue;
const float hue_step_size = hue_diff / (channels - 1);
const float eta_diff = max_eta - min_eta;
const float eta_step_size = eta_diff / (channels - 1);
const int num_samples = 4;
vec3 grand_color = vec3(0.0);
for(int s = 0; s < num_samples; s++)
{
float curr_hue = min_hue;
float curr_eta = min_eta;
vec3 color = vec3(0.0);
vec4 rand_origin = origin + vec4(RandomUnitVector(prng_state), 0.0)*0.01;
vec4 rand_direction = focal_point_on_plane - rand_origin;
for(int c = 0; c < channels; c++, curr_hue += hue_step_size, curr_eta += eta_step_size)
{
const float f = get_ray0(rand_origin, rand_direction, curr_hue, curr_eta);
const vec3 mask = hsv2rgb(vec3(curr_hue, 1.0, 1.0));
color += f*mask;
}
grand_color += color;
}
grand_color /= num_samples;
//color = pow(color, vec3(2.2));
imageStore(color_image, ivec2(gl_LaunchIDEXT.xy), vec4(grand_color, 1.0));
}
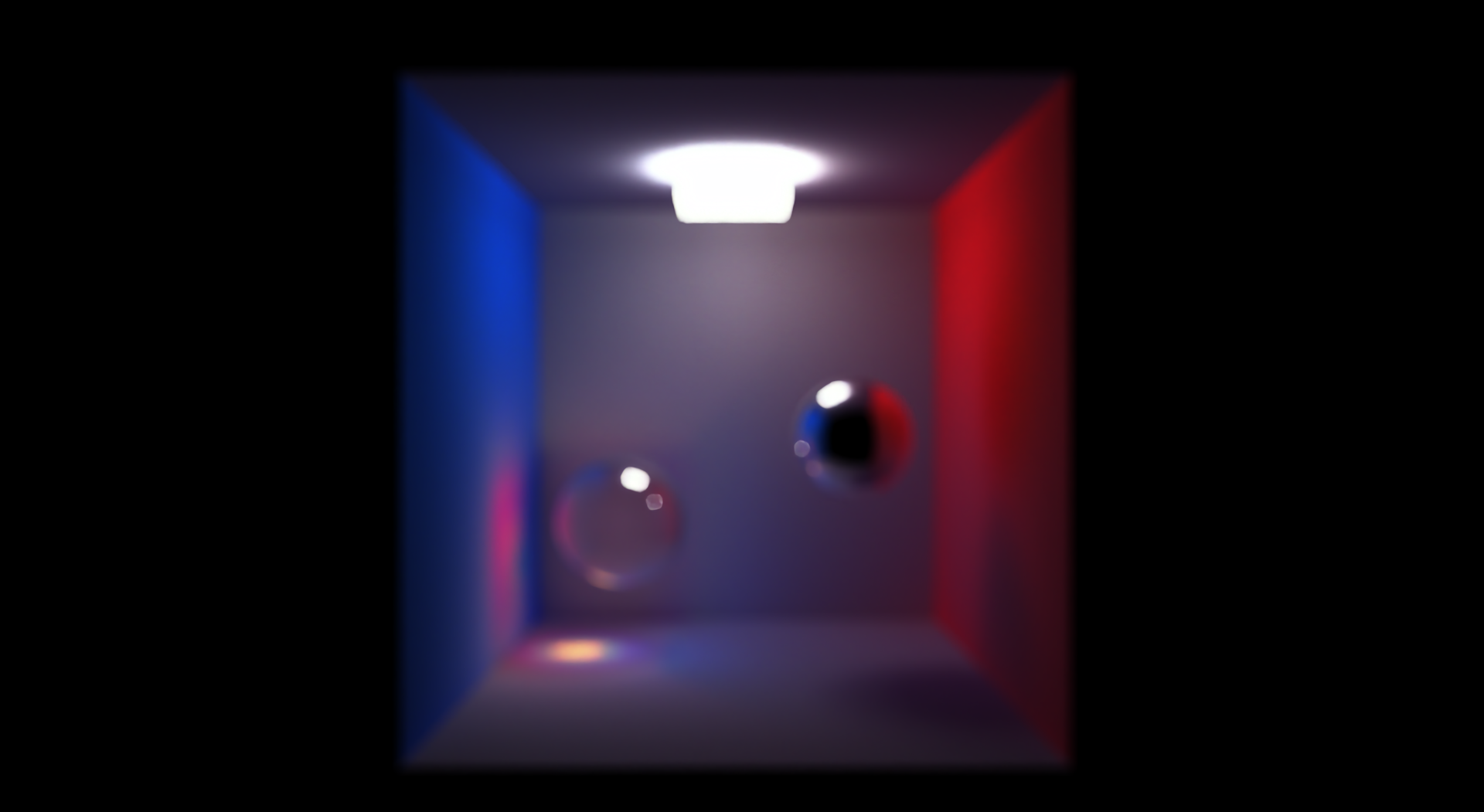
Yeah, pretty simple.
So currently you form a cone with the tip at the camera / eye.
Now real lenses have some effect of ‘crossing’ at a distance, and only this region is sharp.
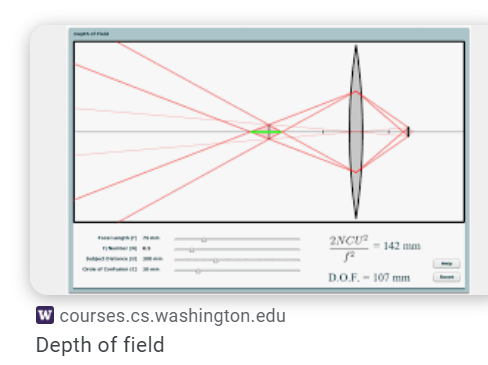
I do not really grasp this, but i guess it's simple as well.